Asymptotic Stability of a Flying Wing in Cruise Trimmed Conditions¶
A Horten flying wing is analysed. The nonlinear trim condition is found and the system is linearised. The eigenvalues of the linearised system are then used to evaluate the stability at the cruise trimmed flight conditions.
[1]:
# required packages
import sharpy.utils.algebra as algebra
import sharpy.sharpy_main
from cases.hangar.richards_wing import Baseline
import numpy as np
import configobj
import matplotlib.pyplot as plt
Flight Conditions¶
Initial flight conditions. The values for angle of attack alpha
, control surface deflection cs_deflection
and thrust
are only initial values. The values required for trim will be calculated by the StaticTrim
routine
[2]:
u_inf = 28
alpha_deg = 4.5135
cs_deflection = 0.1814
thrust = 5.5129
Discretisation¶
[3]:
M = 4 # chordwise panels
N = 11 # spanwise panels
Msf = 5 # wake length in chord numbers
Create Horten Wing¶
[4]:
ws = Baseline(M=M,
N=N,
Mstarfactor=Msf,
u_inf=u_inf,
rho=1.02,
alpha_deg=alpha_deg,
roll_deg=0,
cs_deflection_deg=cs_deflection,
thrust=thrust,
physical_time=20,
case_name='horten',
case_name_format=4,
case_remarks='M%gN%gMsf%g' % (M, N, Msf))
ws.set_properties()
ws.initialise()
ws.clean_test_files()
ws.update_mass_stiffness(sigma=1., sigma_mass=2.5)
ws.update_fem_prop()
ws.generate_fem_file()
ws.update_aero_properties()
ws.generate_aero_file()
0
Section Mass: 11.88
Linear Mass: 11.88
Section Ixx: 1.8777
Section Iyy: 1.0137
Section Izz: 2.5496
Linear Ixx: 1.88
1
Section Mass: 10.99
Linear Mass: 10.99
Section Ixx: 1.4694
Section Iyy: 0.9345
Section Izz: 2.1501
Linear Ixx: 1.74
2
Section Mass: 10.10
Linear Mass: 10.10
Section Ixx: 1.1257
Section Iyy: 0.8561
Section Izz: 1.7993
Linear Ixx: 1.60
3
Section Mass: 9.21
Linear Mass: 9.21
Section Ixx: 0.8410
Section Iyy: 0.7783
Section Izz: 1.4933
Linear Ixx: 1.46
4
Section Mass: 8.32
Linear Mass: 8.32
Section Ixx: 0.6096
Section Iyy: 0.7011
Section Izz: 1.2280
Linear Ixx: 1.31
5
Section Mass: 7.43
Linear Mass: 7.43
Section Ixx: 0.4260
Section Iyy: 0.6246
Section Izz: 0.9996
Linear Ixx: 1.17
6
Section Mass: 6.54
Linear Mass: 6.54
Section Ixx: 0.2845
Section Iyy: 0.5485
Section Izz: 0.8040
Linear Ixx: 1.03
7
Section Mass: 5.64
Linear Mass: 5.64
Section Ixx: 0.1796
Section Iyy: 0.4728
Section Izz: 0.6374
Linear Ixx: 0.89
8
Section Mass: 4.75
Linear Mass: 4.75
Section Ixx: 0.1055
Section Iyy: 0.3975
Section Izz: 0.4959
Linear Ixx: 0.75
9
Section Mass: 3.86
Linear Mass: 3.86
Section Ixx: 0.0567
Section Iyy: 0.3226
Section Izz: 0.3753
Linear Ixx: 0.61
10
Section Mass: 2.97
Linear Mass: 2.97
Section Ixx: 0.0275
Section Iyy: 0.2479
Section Izz: 0.2719
Linear Ixx: 0.47
Simulation Information¶
The flow
setting tells SHARPy which solvers to run and in which order. You may be stranged by the presence of the DynamicCoupled
solver but it is necessary to give an initial speed to the structure. This will allow proper linearisation of the structural and rigid body equations.
[5]:
flow = ['BeamLoader',
'AerogridLoader',
'StaticTrim',
'BeamPlot',
'AerogridPlot',
'AeroForcesCalculator',
'DynamicCoupled',
'Modal',
'LinearAssembler',
'AsymptoticStability',
'StabilityDerivatives',
]
SHARPy Settings¶
[6]:
settings = dict()
settings['SHARPy'] = {'case': ws.case_name,
'route': ws.case_route,
'flow': flow,
'write_screen': 'on',
'write_log': 'on',
'log_folder': './output/' + ws.case_name + '/',
'log_file': ws.case_name + '.log'}
Loaders¶
[7]:
settings['BeamLoader'] = {'unsteady': 'off',
'orientation': algebra.euler2quat(np.array([ws.roll,
ws.alpha,
ws.beta]))}
settings['AerogridLoader'] = {'unsteady': 'off',
'aligned_grid': 'on',
'mstar': int(ws.M * ws.Mstarfactor),
'freestream_dir': ['1', '0', '0'],
'control_surface_deflection': ['']}
StaticCoupled Solver¶
[8]:
settings['StaticCoupled'] = {'print_info': 'on',
'structural_solver': 'NonLinearStatic',
'structural_solver_settings': {'print_info': 'off',
'max_iterations': 200,
'num_load_steps': 1,
'delta_curved': 1e-5,
'min_delta': ws.tolerance,
'gravity_on': 'on',
'gravity': 9.81},
'aero_solver': 'StaticUvlm',
'aero_solver_settings': {'print_info': 'on',
'horseshoe': ws.horseshoe,
'num_cores': 4,
'n_rollup': int(0),
'rollup_dt': ws.dt,
'rollup_aic_refresh': 1,
'rollup_tolerance': 1e-4,
'velocity_field_generator': 'SteadyVelocityField',
'velocity_field_input': {'u_inf': ws.u_inf,
'u_inf_direction': [1., 0, 0]},
'rho': ws.rho},
'max_iter': 200,
'n_load_steps': 1,
'tolerance': ws.tolerance,
'relaxation_factor': 0.2}
Trim solver¶
[9]:
settings['StaticTrim'] = {'solver': 'StaticCoupled',
'solver_settings': settings['StaticCoupled'],
'thrust_nodes': ws.thrust_nodes,
'initial_alpha': ws.alpha,
'initial_deflection': ws.cs_deflection,
'initial_thrust': ws.thrust,
'max_iter': 200,
'fz_tolerance': 1e-2,
'fx_tolerance': 1e-2,
'm_tolerance': 1e-2}
Nonlinear Equilibrium Post-process¶
[10]:
settings['AerogridPlot'] = {'folder': './output/',
'include_rbm': 'off',
'include_applied_forces': 'on',
'minus_m_star': 0,
'u_inf': ws.u_inf
}
settings['AeroForcesCalculator'] = {'folder': './output/',
'write_text_file': 'off',
'text_file_name': ws.case_name + '_aeroforces.csv',
'screen_output': 'on',
'unsteady': 'off',
'coefficients': True,
'q_ref': 0.5 * ws.rho * ws.u_inf ** 2,
'S_ref': 12.809,
}
settings['BeamPlot'] = {'folder': './output/',
'include_rbm': 'on',
'include_applied_forces': 'on',
'include_FoR': 'on'}
DynamicCoupled Solver¶
As mentioned before, a single time step of DynamicCoupled
is required to give the structure the velocity required for the linearisation of the rigid body equations to be correct. Hence n_time_steps = 1
[11]:
struct_solver_settings = {'print_info': 'off',
'initial_velocity_direction': [-1., 0., 0.],
'max_iterations': 950,
'delta_curved': 1e-6,
'min_delta': ws.tolerance,
'newmark_damp': 5e-3,
'gravity_on': True,
'gravity': 9.81,
'num_steps': ws.n_tstep,
'dt': ws.dt,
'initial_velocity': ws.u_inf * 1}
step_uvlm_settings = {'print_info': 'on',
'horseshoe': ws.horseshoe,
'num_cores': 4,
'n_rollup': 1,
'convection_scheme': ws.wake_type,
'rollup_dt': ws.dt,
'rollup_aic_refresh': 1,
'rollup_tolerance': 1e-4,
'velocity_field_generator': 'SteadyVelocityField',
'velocity_field_input': {'u_inf': ws.u_inf * 0,
'u_inf_direction': [1., 0., 0.]},
'rho': ws.rho,
'n_time_steps': ws.n_tstep,
'dt': ws.dt,
'gamma_dot_filtering': 3}
settings['DynamicCoupled'] = {'print_info': 'on',
'structural_solver': 'NonLinearDynamicCoupledStep',
'structural_solver_settings': struct_solver_settings,
'aero_solver': 'StepUvlm',
'aero_solver_settings': step_uvlm_settings,
'fsi_substeps': 200,
'fsi_tolerance': ws.fsi_tolerance,
'relaxation_factor': ws.relaxation_factor,
'minimum_steps': 1,
'relaxation_steps': 150,
'final_relaxation_factor': 0.5,
'n_time_steps': 1,
'dt': ws.dt,
'include_unsteady_force_contribution': 'off',
}
Modal Solver Settings¶
[12]:
settings['Modal'] = {'print_info': True,
'use_undamped_modes': True,
'NumLambda': 30,
'rigid_body_modes': True,
'write_modes_vtk': 'on',
'print_matrices': 'on',
'write_data': 'on',
'continuous_eigenvalues': 'off',
'dt': ws.dt,
'plot_eigenvalues': False,
'rigid_modes_cg': False}
Linear Assembler Settings¶
Note that for the assembly of the linear system, we replace the parametrisation of the orientation with Euler angles instead of quaternions.
[13]:
settings['LinearAssembler'] = {'linear_system': 'LinearAeroelastic',
'linear_system_settings': {
'beam_settings': {'modal_projection': 'off',
'inout_coords': 'modes',
'discrete_time': True,
'newmark_damp': 0.5e-2,
'discr_method': 'newmark',
'dt': ws.dt,
'proj_modes': 'undamped',
'num_modes': 9,
'print_info': 'on',
'gravity': 'on',
'remove_dofs': []},
'aero_settings': {'dt': ws.dt,
'integr_order': 2,
'density': ws.rho,
'remove_predictor': 'off',
'use_sparse': 'off',
'rigid_body_motion': 'on',
'remove_inputs': ['u_gust']},
'rigid_body_motion': True,
'track_body': 'on',
'use_euler': 'on',
'linearisation_tstep': -1
}}
Asymptotic Stability Post-processor¶
[14]:
settings['AsymptoticStability'] = {
'print_info': 'on',
'frequency_cutoff': 0,
'export_eigenvalues': 'on',
'num_evals': 1000,
'folder': './output/'}
Stability Derivatives Post-processor¶
[15]:
settings['StabilityDerivatives'] = {'u_inf': ws.u_inf,
'S_ref': 12.809,
'b_ref': ws.span,
'c_ref': 0.719}
Write solver file¶
[16]:
config = configobj.ConfigObj()
np.set_printoptions(precision=16)
file_name = ws.case_route + '/' + ws.case_name + '.sharpy'
config.filename = file_name
for k, v in settings.items():
config[k] = v
config.write()
Run Simulation¶
[17]:
data = sharpy.sharpy_main.main(['', ws.case_route + '/' + ws.case_name + '.sharpy'])
--------------------------------------------------------------------------------
###### ## ## ### ######## ######## ## ##
## ## ## ## ## ## ## ## ## ## ## ##
## ## ## ## ## ## ## ## ## ####
###### ######### ## ## ######## ######## ##
## ## ## ######### ## ## ## ##
## ## ## ## ## ## ## ## ## ##
###### ## ## ## ## ## ## ## ##
--------------------------------------------------------------------------------
Aeroelastics Lab, Aeronautics Department.
Copyright (c), Imperial College London.
All rights reserved.
License available at https://github.com/imperialcollegelondon/sharpy
Running SHARPy from /home/ng213/code/sharpy/docs/source/content/example_notebooks
SHARPy being run is in /home/ng213/code/sharpy
The branch being run is dev_examples
The version and commit hash are: v0.1-1590-g7385fe4-7385fe4
The available solvers on this session are:
PreSharpy
_BaseStructural
AerogridLoader
BeamLoader
DynamicCoupled
DynamicUVLM
LinDynamicSim
LinearAssembler
Modal
NoAero
NonLinearDynamic
NonLinearDynamicCoupledStep
NonLinearDynamicMultibody
NonLinearDynamicPrescribedStep
NonLinearStatic
NonLinearStaticMultibody
PrescribedUvlm
RigidDynamicPrescribedStep
SHWUvlm
StaticCoupled
StaticCoupledRBM
StaticTrim
StaticUvlm
StepLinearUVLM
StepUvlm
Trim
Cleanup
PickleData
SaveData
CreateSnapshot
PlotFlowField
StabilityDerivatives
AeroForcesCalculator
WriteVariablesTime
AerogridPlot
LiftDistribution
StallCheck
FrequencyResponse
AsymptoticStability
BeamPlot
BeamLoads
Generating an instance of BeamLoader
Generating an instance of AerogridLoader
Variable control_surface_deflection_generator_settings has no assigned value in the settings file.
will default to the value: []
The aerodynamic grid contains 4 surfaces
Surface 0, M=4, N=2
Wake 0, M=20, N=2
Surface 1, M=4, N=22
Wake 1, M=20, N=22
Surface 2, M=4, N=2
Wake 2, M=20, N=2
Surface 3, M=4, N=22
Wake 3, M=20, N=22
In total: 192 bound panels
In total: 960 wake panels
Total number of panels = 1152
Generating an instance of StaticTrim
Variable print_info has no assigned value in the settings file.
will default to the value: c_bool(True)
Variable tail_cs_index has no assigned value in the settings file.
will default to the value: c_int(0)
Variable initial_angle_eps has no assigned value in the settings file.
will default to the value: c_double(0.05)
Variable initial_thrust_eps has no assigned value in the settings file.
will default to the value: c_double(2.0)
Variable relaxation_factor has no assigned value in the settings file.
will default to the value: c_double(0.2)
Generating an instance of StaticCoupled
Generating an instance of NonLinearStatic
Variable newmark_damp has no assigned value in the settings file.
will default to the value: c_double(0.0001)
Variable relaxation_factor has no assigned value in the settings file.
will default to the value: c_double(0.3)
Variable dt has no assigned value in the settings file.
will default to the value: c_double(0.01)
Variable num_steps has no assigned value in the settings file.
will default to the value: c_int(500)
Variable initial_position has no assigned value in the settings file.
will default to the value: [0. 0. 0.]
Generating an instance of StaticUvlm
Variable iterative_solver has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable iterative_tol has no assigned value in the settings file.
will default to the value: c_double(0.0001)
Variable iterative_precond has no assigned value in the settings file.
will default to the value: c_bool(False)
|=====|=====|============|==========|==========|==========|==========|==========|==========|
|iter |step | log10(res) | Fx | Fy | Fz | Mx | My | Mz |
|=====|=====|============|==========|==========|==========|==========|==========|==========|
|==========|==========|==========|==========|==========|==========|==========|==========|==========|==========|
| iter | alpha | elev | thrust | Fx | Fy | Fz | Mx | My | Mz |
|==========|==========|==========|==========|==========|==========|==========|==========|==========|==========|
| 0 | 0 | 0.00000 | -0.1051 | -0.0000 | 0.0604 | -0.0000 | 1.0816 | -0.0000 |
| 1 | 0 | -7.62661 | -0.1119 | 0.0000 | 0.1274 | -0.0000 | 0.0026 | 0.0000 |
| 2 | 0 | -8.34118 | -0.0941 | -0.0000 | 0.0393 | 0.0000 | -0.0793 | 0.0000 |
| 3 | 0 | -9.29266 | -0.0870 | 0.0000 | 0.0007 | -0.0000 | -0.0089 | 0.0000 |
| 4 | 0 | -10.67443 | -0.0876 | 0.0000 | 0.0028 | 0.0000 | -0.0119 | 0.0000 |
| 5 | 0 | -10.87008 | -0.0878 | 0.0000 | 0.0039 | -0.0000 | -0.0138 | 0.0000 |
| 6 | 0 | -11.64423 | -0.0877 | -0.0000 | 0.0037 | 0.0000 | -0.0135 | -0.0000 |
| 7 | 0 | -12.87835 | -0.0877 | 0.0000 | 0.0037 | -0.0000 | -0.0135 | 0.0000 |
| 0 | 4.5135 | 0.1814 | 5.5129 | -0.0877 | 0.0000 | 0.0037 | -0.0000 | -0.0135 | 0.0000 |
| 0 | 0 | 0.00000 |-116.9870 | -0.0000 | 994.8061 | -0.0000 |-882.4096 | 0.0000 |
| 1 | 0 | -5.81338 | -81.0252 | -0.0000 | 944.6090 | -0.0000 |-802.5942 | -0.0000 |
| 2 | 0 | -6.69380 | -74.1802 | -0.0000 | 937.6597 | -0.0000 |-792.1302 | 0.0000 |
| 3 | 0 | -7.20553 | -75.0015 | 0.0000 | 939.7800 | -0.0000 |-795.7138 | -0.0000 |
| 4 | 0 | -8.63165 | -74.9725 | 0.0000 | 939.7033 | -0.0000 |-795.5808 | 0.0000 |
| 5 | 0 | -8.79020 | -74.9511 | 0.0000 | 939.6488 | 0.0000 |-795.4881 | 0.0000 |
| 6 | 0 | -9.56992 | -74.9546 | -0.0000 | 939.6578 | 0.0000 |-795.5035 | -0.0000 |
| 7 | 0 | -10.77969 | -74.9549 | 0.0000 | 939.6584 | -0.0000 |-795.5044 | 0.0000 |
| 8 | 0 | -10.98913 | -74.9547 | -0.0000 | 939.6580 | 0.0000 |-795.5039 | -0.0000 |
| 9 | 0 | -12.12706 | -74.9547 | -0.0000 | 939.6581 | 0.0000 |-795.5039 | -0.0000 |
| 0 | 7.3783 | -2.6834 | 5.5129 | -74.9547 | -0.0000 | 939.6581 | 0.0000 |-795.5039 | -0.0000 |
| 0 | 0 | 0.00000 | -32.9132 | -0.0000 | 371.4719 | -0.0000 |-902.7965 | -0.0000 |
| 1 | 0 | -5.48782 | -11.8207 | 0.0000 | 298.8468 | -0.0000 |-777.2958 | 0.0000 |
| 2 | 0 | -6.40702 | -8.5206 | 0.0000 | 289.8069 | -0.0000 |-761.4879 | -0.0000 |
| 3 | 0 | -6.85006 | -9.2671 | -0.0000 | 293.2383 | 0.0000 |-767.3401 | -0.0000 |
| 4 | 0 | -8.25284 | -9.2365 | 0.0000 | 293.1025 | -0.0000 |-767.1091 | 0.0000 |
| 5 | 0 | -8.43408 | -9.2166 | 0.0000 | 293.0132 | 0.0000 |-766.9570 | 0.0000 |
| 6 | 0 | -9.20313 | -9.2199 | -0.0000 | 293.0284 | -0.0000 |-766.9829 | -0.0000 |
| 7 | 0 | -10.44114 | -9.2201 | 0.0000 | 293.0293 | -0.0000 |-766.9844 | 0.0000 |
| 8 | 0 | -10.62337 | -9.2200 | -0.0000 | 293.0287 | 0.0000 |-766.9834 | -0.0000 |
| 9 | 0 | -11.73764 | -9.2200 | -0.0000 | 293.0287 | 0.0000 |-766.9835 | -0.0000 |
| 10 | 0 | -12.29756 | -9.2200 | 0.0000 | 293.0287 | -0.0000 |-766.9835 | 0.0000 |
| 0 | 4.5135 | 3.0462 | 5.5129 | -9.2200 | 0.0000 | 293.0287 | -0.0000 |-766.9835 | 0.0000 |
| 0 | 0 | 0.00000 | -4.1051 | -0.0000 | 0.0604 | -0.0000 | 1.0812 | -0.0000 |
| 1 | 0 | -7.62660 | -4.1120 | -0.0000 | 0.1274 | -0.0000 | 0.0023 | -0.0000 |
| 2 | 0 | -8.34116 | -4.0942 | -0.0000 | 0.0393 | 0.0000 | -0.0796 | -0.0000 |
| 3 | 0 | -9.29268 | -4.0871 | 0.0000 | 0.0007 | -0.0000 | -0.0093 | 0.0000 |
| 4 | 0 | -10.67446 | -4.0876 | 0.0000 | 0.0028 | 0.0000 | -0.0123 | 0.0000 |
| 5 | 0 | -10.86979 | -4.0878 | 0.0000 | 0.0039 | -0.0000 | -0.0142 | 0.0000 |
| 6 | 0 | -11.64229 | -4.0878 | 0.0000 | 0.0037 | -0.0000 | -0.0138 | 0.0000 |
| 7 | 0 | -12.86703 | -4.0878 | 0.0000 | 0.0037 | -0.0000 | -0.0138 | 0.0000 |
| 0 | 4.5135 | 0.1814 | 7.5129 | -4.0878 | 0.0000 | 0.0037 | -0.0000 | -0.0138 | 0.0000 |
| 0 | 0 | 0.00000 | -0.0165 | 0.0000 | 0.0499 | 0.0000 | 1.1009 | 0.0000 |
| 1 | 0 | -7.62629 | -0.0236 | 0.0000 | 0.1184 | 0.0000 | 0.0195 | 0.0000 |
| 2 | 0 | -8.34096 | -0.0058 | -0.0000 | 0.0305 | -0.0000 | -0.0628 | -0.0000 |
| 3 | 0 | -9.29199 | 0.0012 | 0.0000 | -0.0082 | 0.0000 | 0.0077 | 0.0000 |
| 4 | 0 | -10.67407 | 0.0007 | 0.0000 | -0.0061 | -0.0000 | 0.0047 | 0.0000 |
| 5 | 0 | -10.86912 | 0.0005 | -0.0000 | -0.0050 | 0.0000 | 0.0028 | -0.0000 |
| 6 | 0 | -11.64225 | 0.0005 | 0.0000 | -0.0052 | -0.0000 | 0.0031 | 0.0000 |
| 7 | 0 | -12.83721 | 0.0005 | -0.0000 | -0.0052 | 0.0000 | 0.0032 | -0.0000 |
| 1 | 4.5135 | 0.1814 | 5.4690 | 0.0005 | -0.0000 | -0.0052 | 0.0000 | 0.0032 | -0.0000 |
Generating an instance of BeamPlot
Variable include_applied_moments has no assigned value in the settings file.
will default to the value: c_bool(True)
Variable name_prefix has no assigned value in the settings file.
will default to the value:
Variable output_rbm has no assigned value in the settings file.
will default to the value: c_bool(True)
...Finished
Generating an instance of AerogridPlot
Variable include_forward_motion has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable include_unsteady_applied_forces has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable name_prefix has no assigned value in the settings file.
will default to the value:
Variable dt has no assigned value in the settings file.
will default to the value: c_double(0.0)
Variable include_velocities has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable num_cores has no assigned value in the settings file.
will default to the value: c_int(1)
...Finished
Generating an instance of AeroForcesCalculator
--------------------------------------------------------------------------------
tstep | fx_g | fy_g | fz_g | Cfx_g | Cfy_g | Cfz_g
0 | 1.090e+01 | -1.250e-07 | 1.835e+03 | 2.129e-03 | -2.441e-11 | 3.583e-01
...Finished
Generating an instance of DynamicCoupled
Variable structural_substeps has no assigned value in the settings file.
will default to the value: c_int(0)
Variable dynamic_relaxation has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable postprocessors has no assigned value in the settings file.
will default to the value: []
Variable postprocessors_settings has no assigned value in the settings file.
will default to the value: {}
Variable controller_id has no assigned value in the settings file.
will default to the value: {}
Variable controller_settings has no assigned value in the settings file.
will default to the value: {}
Variable cleanup_previous_solution has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable steps_without_unsteady_force has no assigned value in the settings file.
will default to the value: c_int(0)
Variable pseudosteps_ramp_unsteady_force has no assigned value in the settings file.
will default to the value: c_int(0)
Generating an instance of NonLinearDynamicCoupledStep
Variable num_load_steps has no assigned value in the settings file.
will default to the value: c_int(1)
Variable relaxation_factor has no assigned value in the settings file.
will default to the value: c_double(0.3)
Variable balancing has no assigned value in the settings file.
will default to the value: c_bool(False)
Generating an instance of StepUvlm
Variable iterative_solver has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable iterative_tol has no assigned value in the settings file.
will default to the value: c_double(0.0001)
Variable iterative_precond has no assigned value in the settings file.
will default to the value: c_bool(False)
|=======|========|======|==============|==============|==============|==============|==============|
| ts | t | iter | struc ratio | iter time | residual vel | FoR_vel(x) | FoR_vel(z) |
|=======|========|======|==============|==============|==============|==============|==============|
/home/ng213/code/sharpy/sharpy/aero/utils/uvlmlib.py:230: RuntimeWarning: invalid value encountered in true_divide
flightconditions.uinf_direction = np.ctypeslib.as_ctypes(ts_info.u_ext[0][:, 0, 0]/flightconditions.uinf)
| 1 | 0.0089 | 3 | 0.652648 | 0.921936 | -10.549271 |-2.791317e+01 |-2.203427e+00 |
...Finished
Generating an instance of Modal
Variable folder has no assigned value in the settings file.
will default to the value: ./output
Variable keep_linear_matrices has no assigned value in the settings file.
will default to the value: c_bool(True)
Variable write_dat has no assigned value in the settings file.
will default to the value: c_bool(True)
Variable delta_curved has no assigned value in the settings file.
will default to the value: c_double(0.01)
Variable max_rotation_deg has no assigned value in the settings file.
will default to the value: c_double(15.0)
Variable max_displacement has no assigned value in the settings file.
will default to the value: c_double(0.15)
Variable use_custom_timestep has no assigned value in the settings file.
will default to the value: c_int(-1)
Structural eigenvalues
|==============|==============|==============|==============|==============|==============|==============|
| mode | eval_real | eval_imag | freq_n (Hz) | freq_d (Hz) | damping | period (s) |
|==============|==============|==============|==============|==============|==============|==============|
/home/ng213/code/sharpy/sharpy/solvers/modal.py:284: UserWarning: Projecting a system with damping on undamped modal shapes
'Projecting a system with damping on undamped modal shapes')
| 0 | -0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 1 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 2 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 3 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 4 | -0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 5 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 6 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 7 | -0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 8 | -0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 9 | -0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 10 | 0.000000 | 28.293939 | 4.503120 | 4.503120 | -0.000000 | 0.222068 |
| 11 | 0.000000 | 29.271318 | 4.658675 | 4.658675 | -0.000000 | 0.214653 |
| 12 | 0.000000 | 54.780234 | 8.718545 | 8.718545 | -0.000000 | 0.114698 |
| 13 | 0.000000 | 58.999779 | 9.390106 | 9.390106 | -0.000000 | 0.106495 |
| 14 | 0.000000 | 70.520741 | 11.223724 | 11.223724 | -0.000000 | 0.089097 |
| 15 | 0.000000 | 76.917111 | 12.241738 | 12.241738 | -0.000000 | 0.081688 |
| 16 | 0.000000 | 87.324076 | 13.898058 | 13.898058 | -0.000000 | 0.071952 |
| 17 | 0.000000 | 108.035577 | 17.194396 | 17.194396 | -0.000000 | 0.058158 |
| 18 | 0.000000 | 119.692139 | 19.049596 | 19.049596 | -0.000000 | 0.052495 |
| 19 | 0.000000 | 133.495187 | 21.246419 | 21.246419 | -0.000000 | 0.047067 |
| 20 | 0.000000 | 134.444788 | 21.397553 | 21.397553 | -0.000000 | 0.046734 |
| 21 | 0.000000 | 151.060442 | 24.042016 | 24.042016 | -0.000000 | 0.041594 |
| 22 | 0.000000 | 159.369020 | 25.364367 | 25.364367 | -0.000000 | 0.039425 |
| 23 | 0.000000 | 171.256102 | 27.256255 | 27.256255 | -0.000000 | 0.036689 |
| 24 | 0.000000 | 173.895881 | 27.676389 | 27.676389 | -0.000000 | 0.036132 |
| 25 | 0.000000 | 199.016557 | 31.674469 | 31.674469 | -0.000000 | 0.031571 |
| 26 | 0.000000 | 205.412581 | 32.692428 | 32.692428 | -0.000000 | 0.030588 |
| 27 | 0.000000 | 205.419531 | 32.693534 | 32.693534 | -0.000000 | 0.030587 |
| 28 | 0.000000 | 223.563796 | 35.581283 | 35.581283 | -0.000000 | 0.028105 |
| 29 | 0.000000 | 227.924750 | 36.275351 | 36.275351 | -0.000000 | 0.027567 |
Generating an instance of LinearAssembler
Variable linearisation_tstep has no assigned value in the settings file.
will default to the value: c_int(-1)
Generating an instance of LinearAeroelastic
Variable uvlm_filename has no assigned value in the settings file.
will default to the value:
Generating an instance of LinearUVLM
Variable ScalingDict has no assigned value in the settings file.
will default to the value: {}
Variable gust_assembler has no assigned value in the settings file.
will default to the value:
Variable rom_method has no assigned value in the settings file.
will default to the value: []
Variable rom_method_settings has no assigned value in the settings file.
will default to the value: {}
Variable length has no assigned value in the settings file.
will default to the value: 1.0
Variable speed has no assigned value in the settings file.
will default to the value: 1.0
Variable density has no assigned value in the settings file.
will default to the value: 1.0
Initialising Static linear UVLM solver class...
...done in 0.27 sec
State-space realisation of UVLM equations started...
state-space model produced in form:
x_{n+1} = A x_{n} + Bp u_{n+1}
...done in 2.44 sec
Generating an instance of LinearBeam
Variable remove_sym_modes has no assigned value in the settings file.
will default to the value: False
Warning, projecting system with damping onto undamped modes
Linearising gravity terms...
M = 187.12 kg
X_CG A -> 1.19 -0.00 0.01
Node 1 -> B 0.000 -0.089 -0.000
-> A 0.089 0.206 0.000
-> G 0.089 0.206 -0.007
Node mass:
Matrix: 2.6141
Node 2 -> B -0.010 -0.019 -0.000
-> A 0.019 0.403 0.000
-> G 0.019 0.403 -0.001
Node mass:
Matrix: 7.3672
Node 3 -> B -0.019 -0.087 -0.000
-> A 0.234 0.800 0.000
-> G 0.234 0.800 -0.018
Node mass:
Matrix: 5.8780
Node 4 -> B -0.019 -0.084 -0.000
-> A 0.390 1.238 0.001
-> G 0.389 1.238 -0.030
Node mass:
Matrix: 2.8288
Node 5 -> B -0.018 -0.081 -0.000
-> A 0.546 1.676 0.001
-> G 0.544 1.676 -0.041
Node mass:
Matrix: 5.4372
Node 6 -> B -0.017 -0.078 -0.000
-> A 0.702 2.113 0.002
-> G 0.700 2.113 -0.053
Node mass:
Matrix: 2.6084
Node 7 -> B -0.016 -0.074 -0.000
-> A 0.857 2.551 0.003
-> G 0.855 2.551 -0.064
Node mass:
Matrix: 4.9963
Node 8 -> B -0.016 -0.071 -0.000
-> A 1.013 2.988 0.004
-> G 1.010 2.988 -0.076
Node mass:
Matrix: 2.3879
Node 9 -> B -0.015 -0.068 -0.000
-> A 1.169 3.426 0.005
-> G 1.166 3.426 -0.087
Node mass:
Matrix: 4.5555
Node 10 -> B -0.014 -0.065 -0.000
-> A 1.325 3.863 0.006
-> G 1.321 3.863 -0.098
Node mass:
Matrix: 2.1675
Node 11 -> B -0.013 -0.061 -0.000
-> A 1.480 4.301 0.007
-> G 1.476 4.301 -0.109
Node mass:
Matrix: 4.1146
Node 12 -> B -0.013 -0.058 -0.000
-> A 1.636 4.739 0.009
-> G 1.632 4.739 -0.120
Node mass:
Matrix: 1.9471
Node 13 -> B -0.012 -0.055 -0.000
-> A 1.792 5.176 0.010
-> G 1.787 5.176 -0.131
Node mass:
Matrix: 3.6738
Node 14 -> B -0.011 -0.052 -0.000
-> A 1.948 5.614 0.011
-> G 1.943 5.614 -0.142
Node mass:
Matrix: 1.7267
Node 15 -> B -0.011 -0.048 -0.000
-> A 2.104 6.052 0.012
-> G 2.098 6.052 -0.153
Node mass:
Matrix: 3.2329
Node 16 -> B -0.010 -0.045 -0.000
-> A 2.260 6.489 0.014
-> G 2.254 6.489 -0.164
Node mass:
Matrix: 1.5062
Node 17 -> B -0.009 -0.042 -0.000
-> A 2.415 6.927 0.015
-> G 2.409 6.927 -0.175
Node mass:
Matrix: 2.7921
Node 18 -> B -0.008 -0.039 -0.000
-> A 2.571 7.364 0.016
-> G 2.564 7.364 -0.186
Node mass:
Matrix: 1.2858
Node 19 -> B -0.008 -0.035 -0.000
-> A 2.727 7.802 0.017
-> G 2.720 7.802 -0.197
Node mass:
Matrix: 2.3512
Node 20 -> B -0.007 -0.032 -0.000
-> A 2.883 8.239 0.019
-> G 2.875 8.239 -0.208
Node mass:
Matrix: 1.0654
Node 21 -> B -0.006 -0.028 -0.000
-> A 3.038 8.677 0.020
-> G 3.030 8.677 -0.219
Node mass:
Matrix: 1.9104
Node 22 -> B -0.006 -0.026 -0.000
-> A 3.194 9.114 0.021
-> G 3.186 9.114 -0.230
Node mass:
Matrix: 0.8450
Node 23 -> B -0.005 -0.022 -0.000
-> A 3.350 9.552 0.023
-> G 3.341 9.552 -0.241
Node mass:
Matrix: 1.4695
Node 24 -> B -0.005 -0.022 -0.000
-> A 3.508 9.988 0.024
-> G 3.499 9.988 -0.252
Node mass:
Matrix: 0.3674
Node 25 -> B 0.000 0.089 -0.000
-> A 0.089 -0.206 0.000
-> G 0.089 -0.206 -0.007
Node mass:
Matrix: 2.6141
Node 26 -> B -0.010 0.019 -0.000
-> A 0.019 -0.403 0.000
-> G 0.019 -0.403 -0.001
Node mass:
Matrix: 7.3672
Node 27 -> B -0.019 0.087 -0.000
-> A 0.234 -0.800 0.000
-> G 0.234 -0.800 -0.018
Node mass:
Matrix: 5.8780
Node 28 -> B -0.019 0.084 -0.000
-> A 0.390 -1.238 0.001
-> G 0.389 -1.238 -0.030
Node mass:
Matrix: 2.8288
Node 29 -> B -0.018 0.081 -0.000
-> A 0.546 -1.676 0.001
-> G 0.544 -1.676 -0.041
Node mass:
Matrix: 5.4372
Node 30 -> B -0.017 0.078 -0.000
-> A 0.702 -2.113 0.002
-> G 0.700 -2.113 -0.053
Node mass:
Matrix: 2.6084
Node 31 -> B -0.016 0.074 -0.000
-> A 0.857 -2.551 0.003
-> G 0.855 -2.551 -0.064
Node mass:
Matrix: 4.9963
Node 32 -> B -0.016 0.071 -0.000
-> A 1.013 -2.988 0.004
-> G 1.010 -2.988 -0.076
Node mass:
Matrix: 2.3879
Node 33 -> B -0.015 0.068 -0.000
-> A 1.169 -3.426 0.005
-> G 1.166 -3.426 -0.087
Node mass:
Matrix: 4.5555
Node 34 -> B -0.014 0.065 -0.000
-> A 1.325 -3.863 0.006
-> G 1.321 -3.863 -0.098
Node mass:
Matrix: 2.1675
Node 35 -> B -0.013 0.061 -0.000
-> A 1.480 -4.301 0.007
-> G 1.476 -4.301 -0.109
Node mass:
Matrix: 4.1146
Node 36 -> B -0.013 0.058 -0.000
-> A 1.636 -4.739 0.009
-> G 1.632 -4.739 -0.120
Node mass:
Matrix: 1.9471
Node 37 -> B -0.012 0.055 -0.000
-> A 1.792 -5.176 0.010
-> G 1.787 -5.176 -0.131
Node mass:
Matrix: 3.6738
Node 38 -> B -0.011 0.052 -0.000
-> A 1.948 -5.614 0.011
-> G 1.943 -5.614 -0.142
Node mass:
Matrix: 1.7267
Node 39 -> B -0.011 0.048 -0.000
-> A 2.104 -6.052 0.012
-> G 2.098 -6.052 -0.153
Node mass:
Matrix: 3.2329
Node 40 -> B -0.010 0.045 -0.000
-> A 2.260 -6.489 0.014
-> G 2.254 -6.489 -0.164
Node mass:
Matrix: 1.5062
Node 41 -> B -0.009 0.042 -0.000
-> A 2.415 -6.927 0.015
-> G 2.409 -6.927 -0.175
Node mass:
Matrix: 2.7921
Node 42 -> B -0.008 0.039 -0.000
-> A 2.571 -7.364 0.016
-> G 2.564 -7.364 -0.186
Node mass:
Matrix: 1.2858
Node 43 -> B -0.008 0.035 -0.000
-> A 2.727 -7.802 0.017
-> G 2.720 -7.802 -0.197
Node mass:
Matrix: 2.3512
Node 44 -> B -0.007 0.032 -0.000
-> A 2.883 -8.239 0.019
-> G 2.875 -8.239 -0.208
Node mass:
Matrix: 1.0654
Node 45 -> B -0.006 0.028 -0.000
-> A 3.038 -8.677 0.020
-> G 3.030 -8.677 -0.219
Node mass:
Matrix: 1.9104
Node 46 -> B -0.006 0.026 -0.000
-> A 3.194 -9.114 0.021
-> G 3.186 -9.114 -0.230
Node mass:
Matrix: 0.8450
Node 47 -> B -0.005 0.022 -0.000
-> A 3.350 -9.552 0.023
-> G 3.341 -9.552 -0.241
Node mass:
Matrix: 1.4695
Node 48 -> B -0.005 0.022 -0.000
-> A 3.508 -9.988 0.024
-> G 3.499 -9.988 -0.252
Node mass:
Matrix: 0.3674
Updated the beam C, modal C and K matrices with the terms from the
gravity linearisation
Aeroelastic system assembled:
Aerodynamic states: 1536
Structural states: 594
Total states: 2130
Inputs: 893
Outputs: 891
Generating an instance of AsymptoticStability
Variable reference_velocity has no assigned value in the settings file.
will default to the value: c_double(1.0)
Variable display_root_locus has no assigned value in the settings file.
will default to the value: c_bool(False)
Variable velocity_analysis has no assigned value in the settings file.
will default to the value: []
Variable modes_to_plot has no assigned value in the settings file.
will default to the value: []
Variable postprocessors has no assigned value in the settings file.
will default to the value: []
Variable postprocessors_settings has no assigned value in the settings file.
will default to the value: {}
Dynamical System Eigenvalues
|==============|==============|==============|==============|==============|==============|==============|
| mode | eval_real | eval_imag | freq_n (Hz) | freq_d (Hz) | damping | period (s) |
|==============|==============|==============|==============|==============|==============|==============|
| 0 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 1 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 2 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 3 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 4 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 5 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 6 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 7 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 8 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 9 | -0.000000 | 0.000000 | 0.000000 | 0.000000 | 1.000000 | inf |
| 10 | -0.000884 | 0.321695 | 0.051200 | 0.051199 | 0.002749 | 19.531499 |
| 11 | -0.000884 | -0.321695 | 0.051200 | 0.051199 | 0.002749 | 19.531499 |
| 12 | -0.008471 | -0.391290 | 0.062290 | 0.062276 | 0.021644 | 16.057627 |
| 13 | -0.008471 | 0.391290 | 0.062290 | 0.062276 | 0.021644 | 16.057627 |
| 14 | -0.022506 | 0.000000 | 0.003582 | 0.000000 | 1.000000 | inf |
| 15 | -0.064807 | -53.732340 | 8.551774 | 8.551767 | 0.001206 | 0.116935 |
| 16 | -0.064807 | 53.732340 | 8.551774 | 8.551767 | 0.001206 | 0.116935 |
| 17 | -0.101946 | 68.319126 | 10.873339 | 10.873327 | 0.001492 | 0.091968 |
| 18 | -0.101946 | -68.319126 | 10.873339 | 10.873327 | 0.001492 | 0.091968 |
| 19 | -0.147587 | 83.265087 | 13.252071 | 13.252050 | 0.001772 | 0.075460 |
| 20 | -0.147587 | -83.265087 | 13.252071 | 13.252050 | 0.001772 | 0.075460 |
| 21 | -0.248703 | 109.925761 | 17.495273 | 17.495228 | 0.002262 | 0.057158 |
| 22 | -0.248703 | -109.925761 | 17.495273 | 17.495228 | 0.002262 | 0.057158 |
| 23 | -0.293471 | -120.387486 | 19.160320 | 19.160263 | 0.002438 | 0.052191 |
| 24 | -0.293471 | 120.387486 | 19.160320 | 19.160263 | 0.002438 | 0.052191 |
| 25 | -0.350319 | -132.903267 | 21.152285 | 21.152212 | 0.002636 | 0.047276 |
| 26 | -0.350319 | 132.903267 | 21.152285 | 21.152212 | 0.002636 | 0.047276 |
| 27 | -0.376400 | -138.516845 | 22.045722 | 22.045641 | 0.002717 | 0.045360 |
| 28 | -0.376400 | 138.516845 | 22.045722 | 22.045641 | 0.002717 | 0.045360 |
| 29 | -0.494445 | -162.714219 | 25.896892 | 25.896772 | 0.003039 | 0.038615 |
| 30 | -0.494445 | 162.714219 | 25.896892 | 25.896772 | 0.003039 | 0.038615 |
| 31 | -0.511650 | 166.238203 | 26.457757 | 26.457632 | 0.003078 | 0.037796 |
| 32 | -0.511650 | -166.238203 | 26.457757 | 26.457632 | 0.003078 | 0.037796 |
| 33 | -0.559180 | 175.709808 | 27.965226 | 27.965084 | 0.003182 | 0.035759 |
| 34 | -0.559180 | -175.709808 | 27.965226 | 27.965084 | 0.003182 | 0.035759 |
| 35 | -0.569755 | -177.873185 | 28.309542 | 28.309397 | 0.003203 | 0.035324 |
| 36 | -0.569755 | 177.873185 | 28.309542 | 28.309397 | 0.003203 | 0.035324 |
| 37 | -0.669914 | -197.999013 | 31.512702 | 31.512522 | 0.003383 | 0.031733 |
| 38 | -0.669914 | 197.999013 | 31.512702 | 31.512522 | 0.003383 | 0.031733 |
| 39 | -0.678424 | -199.782668 | 31.796582 | 31.796399 | 0.003396 | 0.031450 |
| 40 | -0.678424 | 199.782668 | 31.796582 | 31.796399 | 0.003396 | 0.031450 |
| 41 | -0.715684 | -207.440558 | 33.015387 | 33.015190 | 0.003450 | 0.030289 |
| 42 | -0.715684 | 207.440558 | 33.015387 | 33.015190 | 0.003450 | 0.030289 |
| 43 | -0.721193 | -208.622990 | 33.203578 | 33.203380 | 0.003457 | 0.030117 |
| 44 | -0.721193 | 208.622990 | 33.203578 | 33.203380 | 0.003457 | 0.030117 |
| 45 | -0.796838 | -224.809925 | 35.779836 | 35.779611 | 0.003544 | 0.027949 |
| 46 | -0.796838 | 224.809925 | 35.779836 | 35.779611 | 0.003544 | 0.027949 |
| 47 | -0.801462 | -225.851206 | 35.945562 | 35.945336 | 0.003549 | 0.027820 |
| 48 | -0.801462 | 225.851206 | 35.945562 | 35.945336 | 0.003549 | 0.027820 |
| 49 | -0.823221 | -257.880049 | 41.043094 | 41.042885 | 0.003192 | 0.024365 |
| 50 | -0.823221 | 257.880049 | 41.043094 | 41.042885 | 0.003192 | 0.024365 |
| 51 | -0.829849 | 232.223375 | 36.959734 | 36.959498 | 0.003573 | 0.027057 |
| 52 | -0.829849 | -232.223375 | 36.959734 | 36.959498 | 0.003573 | 0.027057 |
| 53 | -0.833132 | 232.986709 | 37.081224 | 37.080986 | 0.003576 | 0.026968 |
| 54 | -0.833132 | -232.986709 | 37.081224 | 37.080986 | 0.003576 | 0.026968 |
| 55 | -0.837695 | 252.830753 | 40.239485 | 40.239264 | 0.003313 | 0.024851 |
| 56 | -0.837695 | -252.830753 | 40.239485 | 40.239264 | 0.003313 | 0.024851 |
| 57 | -0.843057 | 274.636583 | 43.709976 | 43.709770 | 0.003070 | 0.022878 |
| 58 | -0.843057 | -274.636583 | 43.709976 | 43.709770 | 0.003070 | 0.022878 |
| 59 | -0.855992 | 264.468482 | 42.091687 | 42.091466 | 0.003237 | 0.023758 |
| 60 | -0.855992 | -264.468482 | 42.091687 | 42.091466 | 0.003237 | 0.023758 |
| 61 | -0.864725 | 271.184097 | 43.160509 | 43.160289 | 0.003189 | 0.023169 |
| 62 | -0.864725 | -271.184097 | 43.160509 | 43.160289 | 0.003189 | 0.023169 |
| 63 | -0.871326 | -283.421753 | 45.108186 | 45.107973 | 0.003074 | 0.022169 |
| 64 | -0.871326 | 283.421753 | 45.108186 | 45.107973 | 0.003074 | 0.022169 |
| 65 | -0.878446 | -267.336880 | 42.548216 | 42.547986 | 0.003286 | 0.023503 |
| 66 | -0.878446 | 267.336880 | 42.548216 | 42.547986 | 0.003286 | 0.023503 |
| 67 | -0.882869 | -280.833492 | 44.696259 | 44.696038 | 0.003144 | 0.022373 |
| 68 | -0.882869 | 280.833492 | 44.696259 | 44.696038 | 0.003144 | 0.022373 |
| 69 | -0.884024 | 245.027541 | 38.997598 | 38.997344 | 0.003608 | 0.025643 |
| 70 | -0.884024 | -245.027541 | 38.997598 | 38.997344 | 0.003608 | 0.025643 |
| 71 | -0.886589 | -245.661872 | 39.098556 | 39.098301 | 0.003609 | 0.025577 |
| 72 | -0.886589 | 245.661872 | 39.098556 | 39.098301 | 0.003609 | 0.025577 |
| 73 | -0.891211 | 288.915187 | 45.982499 | 45.982280 | 0.003085 | 0.021748 |
| 74 | -0.891211 | -288.915187 | 45.982499 | 45.982280 | 0.003085 | 0.021748 |
| 75 | -0.908699 | 251.206722 | 39.981053 | 39.980792 | 0.003617 | 0.025012 |
| 76 | -0.908699 | -251.206722 | 39.981053 | 39.980792 | 0.003617 | 0.025012 |
| 77 | -0.910251 | 251.606123 | 40.044620 | 40.044358 | 0.003618 | 0.024972 |
| 78 | -0.910251 | -251.606123 | 40.044620 | 40.044358 | 0.003618 | 0.024972 |
| 79 | -0.914189 | -241.156654 | 38.381549 | 38.381274 | 0.003791 | 0.026054 |
| 80 | -0.914189 | 241.156654 | 38.381549 | 38.381274 | 0.003791 | 0.026054 |
| 81 | -0.915396 | 290.517028 | 46.237451 | 46.237221 | 0.003151 | 0.021628 |
| 82 | -0.915396 | -290.517028 | 46.237451 | 46.237221 | 0.003151 | 0.021628 |
| 83 | -0.933975 | 278.955366 | 44.397374 | 44.397125 | 0.003348 | 0.022524 |
| 84 | -0.933975 | -278.955366 | 44.397374 | 44.397125 | 0.003348 | 0.022524 |
| 85 | -0.943144 | 260.320871 | 41.431625 | 41.431353 | 0.003623 | 0.024136 |
| 86 | -0.943144 | -260.320871 | 41.431625 | 41.431353 | 0.003623 | 0.024136 |
| 87 | -0.944542 | 260.700477 | 41.492042 | 41.491770 | 0.003623 | 0.024101 |
| 88 | -0.944542 | -260.700477 | 41.492042 | 41.491770 | 0.003623 | 0.024101 |
| 89 | -0.953005 | 294.814043 | 46.921357 | 46.921112 | 0.003233 | 0.021312 |
| 90 | -0.953005 | -294.814043 | 46.921357 | 46.921112 | 0.003233 | 0.021312 |
| 91 | -0.960628 | 295.652741 | 47.054843 | 47.054595 | 0.003249 | 0.021252 |
| 92 | -0.960628 | -295.652741 | 47.054843 | 47.054595 | 0.003249 | 0.021252 |
| 93 | -0.960976 | 265.315973 | 42.226626 | 42.226349 | 0.003622 | 0.023682 |
| 94 | -0.960976 | -265.315973 | 42.226626 | 42.226349 | 0.003622 | 0.023682 |
| 95 | -0.961740 | -300.017780 | 47.749558 | 47.749313 | 0.003206 | 0.020943 |
| 96 | -0.961740 | 300.017780 | 47.749558 | 47.749313 | 0.003206 | 0.020943 |
| 97 | -0.961940 | -265.596058 | 42.271203 | 42.270926 | 0.003622 | 0.023657 |
| 98 | -0.961940 | 265.596058 | 42.271203 | 42.270926 | 0.003622 | 0.023657 |
| 99 | -0.965384 | 266.582845 | 42.428256 | 42.427978 | 0.003621 | 0.023569 |
| 100 | -0.965384 | -266.582845 | 42.428256 | 42.427978 | 0.003621 | 0.023569 |
| 101 | -0.968899 | -235.916658 | 37.547619 | 37.547302 | 0.004107 | 0.026633 |
| 102 | -0.968899 | 235.916658 | 37.547619 | 37.547302 | 0.004107 | 0.026633 |
| 103 | -0.969126 | 301.069959 | 47.917020 | 47.916772 | 0.003219 | 0.020870 |
| 104 | -0.969126 | -301.069959 | 47.917020 | 47.916772 | 0.003219 | 0.020870 |
| 105 | -0.977774 | -281.665806 | 44.828775 | 44.828505 | 0.003471 | 0.022307 |
| 106 | -0.977774 | 281.665806 | 44.828775 | 44.828505 | 0.003471 | 0.022307 |
| 107 | -0.984431 | -272.268138 | 43.333103 | 43.332820 | 0.003616 | 0.023077 |
| 108 | -0.984431 | 272.268138 | 43.333103 | 43.332820 | 0.003616 | 0.023077 |
| 109 | -0.985003 | 251.399896 | 40.011843 | 40.011536 | 0.003918 | 0.024993 |
| 110 | -0.985003 | -251.399896 | 40.011843 | 40.011536 | 0.003918 | 0.024993 |
| 111 | -0.985198 | -272.494340 | 43.369105 | 43.368821 | 0.003615 | 0.023058 |
| 112 | -0.985198 | 272.494340 | 43.369105 | 43.368821 | 0.003615 | 0.023058 |
| 113 | -0.998111 | 276.558228 | 44.015896 | 44.015609 | 0.003609 | 0.022719 |
| 114 | -0.998111 | -276.558228 | 44.015896 | 44.015609 | 0.003609 | 0.022719 |
| 115 | -0.998802 | 276.771420 | 44.049826 | 44.049540 | 0.003609 | 0.022702 |
| 116 | -0.998802 | -276.771420 | 44.049826 | 44.049540 | 0.003609 | 0.022702 |
| 117 | -1.002609 | -296.732610 | 47.226731 | 47.226462 | 0.003379 | 0.021175 |
| 118 | -1.002609 | 296.732610 | 47.226731 | 47.226462 | 0.003379 | 0.021175 |
| 119 | -1.006593 | -246.344800 | 39.207320 | 39.206993 | 0.004086 | 0.025506 |
| 120 | -1.006593 | 246.344800 | 39.207320 | 39.206993 | 0.004086 | 0.025506 |
| 121 | -1.012564 | -297.793229 | 47.395538 | 47.395264 | 0.003400 | 0.021099 |
| 122 | -1.012564 | 297.793229 | 47.395538 | 47.395264 | 0.003400 | 0.021099 |
| 123 | -1.014283 | 306.354455 | 48.758093 | 48.757826 | 0.003311 | 0.020510 |
| 124 | -1.014283 | -306.354455 | 48.758093 | 48.757826 | 0.003311 | 0.020510 |
| 125 | -1.014361 | 281.941928 | 44.872742 | 44.872451 | 0.003598 | 0.022285 |
| 126 | -1.014361 | -281.941928 | 44.872742 | 44.872451 | 0.003598 | 0.022285 |
| 127 | -1.014860 | 282.102785 | 44.898343 | 44.898053 | 0.003597 | 0.022273 |
| 128 | -1.014860 | -282.102785 | 44.898343 | 44.898053 | 0.003597 | 0.022273 |
| 129 | -1.017175 | -306.227153 | 48.737834 | 48.737565 | 0.003322 | 0.020518 |
| 130 | -1.017175 | 306.227153 | 48.737834 | 48.737565 | 0.003322 | 0.020518 |
| 131 | -1.017450 | 57.533524 | 9.158176 | 9.156745 | 0.017682 | 0.109209 |
| 132 | -1.017450 | -57.533524 | 9.158176 | 9.156745 | 0.017682 | 0.109209 |
| 133 | -1.021012 | 310.599833 | 49.433766 | 49.433499 | 0.003287 | 0.020229 |
| 134 | -1.021012 | -310.599833 | 49.433766 | 49.433499 | 0.003287 | 0.020229 |
| 135 | -1.021452 | 310.492397 | 49.416667 | 49.416400 | 0.003290 | 0.020236 |
| 136 | -1.021452 | -310.492397 | 49.416667 | 49.416400 | 0.003290 | 0.020236 |
| 137 | -1.022875 | -284.908060 | 45.344818 | 45.344526 | 0.003590 | 0.022053 |
| 138 | -1.022875 | 284.908060 | 45.344818 | 45.344526 | 0.003590 | 0.022053 |
| 139 | -1.023294 | 285.048653 | 45.367195 | 45.366902 | 0.003590 | 0.022043 |
| 140 | -1.023294 | -285.048653 | 45.367195 | 45.366902 | 0.003590 | 0.022043 |
| 141 | -1.036388 | -289.874565 | 46.135265 | 46.134970 | 0.003575 | 0.021676 |
| 142 | -1.036388 | 289.874565 | 46.135265 | 46.134970 | 0.003575 | 0.021676 |
| 143 | -1.036747 | -290.000394 | 46.155291 | 46.154996 | 0.003575 | 0.021666 |
| 144 | -1.036747 | 290.000394 | 46.155291 | 46.154996 | 0.003575 | 0.021666 |
| 145 | -1.040407 | 291.418922 | 46.381058 | 46.380762 | 0.003570 | 0.021561 |
| 146 | -1.040407 | -291.418922 | 46.381058 | 46.380762 | 0.003570 | 0.021561 |
| 147 | -1.040753 | 291.545493 | 46.401202 | 46.400906 | 0.003570 | 0.021551 |
| 148 | -1.040753 | -291.545493 | 46.401202 | 46.400906 | 0.003570 | 0.021551 |
| 149 | -1.041948 | 304.815949 | 48.513248 | 48.512965 | 0.003418 | 0.020613 |
| 150 | -1.041948 | -304.815949 | 48.513248 | 48.512965 | 0.003418 | 0.020613 |
| 151 | -1.042964 | -314.673618 | 50.082137 | 50.081862 | 0.003314 | 0.019967 |
| 152 | -1.042964 | 314.673618 | 50.082137 | 50.081862 | 0.003314 | 0.019967 |
| 153 | -1.043286 | -307.905151 | 49.004908 | 49.004627 | 0.003388 | 0.020406 |
| 154 | -1.043286 | 307.905151 | 49.004908 | 49.004627 | 0.003388 | 0.020406 |
| 155 | -1.044327 | 308.342971 | 49.074590 | 49.074308 | 0.003387 | 0.020377 |
| 156 | -1.044327 | -308.342971 | 49.074590 | 49.074308 | 0.003387 | 0.020377 |
| 157 | -1.046009 | -304.875396 | 48.522712 | 48.522426 | 0.003431 | 0.020609 |
| 158 | -1.046009 | 304.875396 | 48.522712 | 48.522426 | 0.003431 | 0.020609 |
| 159 | -1.047589 | 314.548401 | 50.062210 | 50.061933 | 0.003330 | 0.019975 |
| 160 | -1.047589 | -314.548401 | 50.062210 | 50.061933 | 0.003330 | 0.019975 |
| 161 | -1.049126 | -306.882777 | 48.842196 | 48.841911 | 0.003419 | 0.020474 |
| 162 | -1.049126 | 306.882777 | 48.842196 | 48.841911 | 0.003419 | 0.020474 |
| 163 | -1.050232 | 295.362748 | 47.008739 | 47.008441 | 0.003556 | 0.021273 |
| 164 | -1.050232 | -295.362748 | 47.008739 | 47.008441 | 0.003556 | 0.021273 |
| 165 | -1.050553 | -295.497323 | 47.030157 | 47.029860 | 0.003555 | 0.021263 |
| 166 | -1.050553 | 295.497323 | 47.030157 | 47.029860 | 0.003555 | 0.021263 |
| 167 | -1.051548 | -295.907799 | 47.095486 | 47.095189 | 0.003554 | 0.021234 |
| 168 | -1.051548 | 295.907799 | 47.095486 | 47.095189 | 0.003554 | 0.021234 |
| 169 | -1.051948 | 296.064745 | 47.120465 | 47.120168 | 0.003553 | 0.021222 |
| 170 | -1.051948 | -296.064745 | 47.120465 | 47.120168 | 0.003553 | 0.021222 |
| 171 | -1.054264 | -306.923573 | 48.848692 | 48.848404 | 0.003435 | 0.020471 |
| 172 | -1.054264 | 306.923573 | 48.848692 | 48.848404 | 0.003435 | 0.020471 |
| 173 | -1.054520 | -318.465849 | 50.685692 | 50.685414 | 0.003311 | 0.019730 |
| 174 | -1.054520 | 318.465849 | 50.685692 | 50.685414 | 0.003311 | 0.019730 |
| 175 | -1.055288 | -318.515081 | 50.693528 | 50.693250 | 0.003313 | 0.019726 |
| 176 | -1.055288 | 318.515081 | 50.693528 | 50.693250 | 0.003313 | 0.019726 |
| 177 | -1.057440 | 314.897307 | 50.117746 | 50.117463 | 0.003358 | 0.019953 |
| 178 | -1.057440 | -314.897307 | 50.117746 | 50.117463 | 0.003358 | 0.019953 |
| 179 | -1.058199 | -309.920136 | 49.325609 | 49.325322 | 0.003414 | 0.020274 |
| 180 | -1.058199 | 309.920136 | 49.325609 | 49.325322 | 0.003414 | 0.020274 |
| 181 | -1.059262 | 299.214097 | 47.621701 | 47.621403 | 0.003540 | 0.020999 |
| 182 | -1.059262 | -299.214097 | 47.621701 | 47.621403 | 0.003540 | 0.020999 |
| 183 | -1.059504 | -299.314232 | 47.637638 | 47.637340 | 0.003540 | 0.020992 |
| 184 | -1.059504 | 299.314232 | 47.637638 | 47.637340 | 0.003540 | 0.020992 |
| 185 | -1.060555 | -299.787498 | 47.712961 | 47.712662 | 0.003538 | 0.020959 |
| 186 | -1.060555 | 299.787498 | 47.712961 | 47.712662 | 0.003538 | 0.020959 |
| 187 | -1.060658 | -309.965877 | 49.332890 | 49.332602 | 0.003422 | 0.020271 |
| 188 | -1.060658 | 309.965877 | 49.332890 | 49.332602 | 0.003422 | 0.020271 |
| 189 | -1.060760 | 299.897460 | 47.730462 | 47.730163 | 0.003537 | 0.020951 |
| 190 | -1.060760 | -299.897460 | 47.730462 | 47.730163 | 0.003537 | 0.020951 |
| 191 | -1.064660 | 315.237918 | 50.171959 | 50.171673 | 0.003377 | 0.019932 |
| 192 | -1.064660 | -315.237918 | 50.171959 | 50.171673 | 0.003377 | 0.019932 |
| 193 | -1.065948 | -313.067380 | 49.826510 | 49.826221 | 0.003405 | 0.020070 |
| 194 | -1.065948 | 313.067380 | 49.826510 | 49.826221 | 0.003405 | 0.020070 |
| 195 | -1.066182 | 312.991749 | 49.814473 | 49.814184 | 0.003406 | 0.020075 |
| 196 | -1.066182 | -312.991749 | 49.814473 | 49.814184 | 0.003406 | 0.020075 |
| 197 | -1.070206 | 304.267646 | 48.425999 | 48.425700 | 0.003517 | 0.020650 |
| 198 | -1.070206 | -304.267646 | 48.425999 | 48.425700 | 0.003517 | 0.020650 |
| 199 | -1.070296 | 304.308945 | 48.432572 | 48.432273 | 0.003517 | 0.020647 |
| 200 | -1.070296 | -304.308945 | 48.432572 | 48.432273 | 0.003517 | 0.020647 |
| 201 | -1.071416 | -304.858400 | 48.520021 | 48.519721 | 0.003514 | 0.020610 |
| 202 | -1.071416 | 304.858400 | 48.520021 | 48.519721 | 0.003514 | 0.020610 |
| 203 | -1.071537 | -304.918001 | 48.529507 | 48.529207 | 0.003514 | 0.020606 |
| 204 | -1.071537 | 304.918001 | 48.529507 | 48.529207 | 0.003514 | 0.020606 |
| 205 | -1.071722 | -321.513275 | 51.170711 | 51.170427 | 0.003333 | 0.019543 |
| 206 | -1.071722 | 321.513275 | 51.170711 | 51.170427 | 0.003333 | 0.019543 |
| 207 | -1.072773 | -321.531559 | 51.173622 | 51.173337 | 0.003336 | 0.019541 |
| 208 | -1.072773 | 321.531559 | 51.173622 | 51.173337 | 0.003336 | 0.019541 |
| 209 | -1.073011 | 316.543066 | 50.379683 | 50.379394 | 0.003390 | 0.019849 |
| 210 | -1.073011 | -316.543066 | 50.379683 | 50.379394 | 0.003390 | 0.019849 |
| 211 | -1.073122 | -316.594455 | 50.387862 | 50.387573 | 0.003390 | 0.019846 |
| 212 | -1.073122 | 316.594455 | 50.387862 | 50.387573 | 0.003390 | 0.019846 |
| 213 | -1.075633 | 324.572398 | 51.657585 | 51.657302 | 0.003314 | 0.019358 |
| 214 | -1.075633 | -324.572398 | 51.657585 | 51.657302 | 0.003314 | 0.019358 |
| 215 | -1.076300 | -324.607705 | 51.663205 | 51.662921 | 0.003316 | 0.019356 |
| 216 | -1.076300 | 324.607705 | 51.663205 | 51.662921 | 0.003316 | 0.019356 |
| 217 | -1.078724 | 320.140186 | 50.952182 | 50.951893 | 0.003370 | 0.019626 |
| 218 | -1.078724 | -320.140186 | 50.952182 | 50.951893 | 0.003370 | 0.019626 |
| 219 | -1.078925 | 319.933158 | 50.919233 | 50.918944 | 0.003372 | 0.019639 |
| 220 | -1.078925 | -319.933158 | 50.919233 | 50.918944 | 0.003372 | 0.019639 |
| 221 | -1.080029 | 309.288686 | 49.225123 | 49.224823 | 0.003492 | 0.020315 |
| 222 | -1.080029 | -309.288686 | 49.225123 | 49.224823 | 0.003492 | 0.020315 |
| 223 | -1.080630 | 309.618139 | 49.277557 | 49.277257 | 0.003490 | 0.020293 |
| 224 | -1.080630 | -309.618139 | 49.277557 | 49.277257 | 0.003490 | 0.020293 |
| 225 | -1.080975 | 309.794320 | 49.305598 | 49.305297 | 0.003489 | 0.020282 |
| 226 | -1.080975 | -309.794320 | 49.305598 | 49.305297 | 0.003489 | 0.020282 |
| 227 | -1.081038 | 309.828767 | 49.311080 | 49.310780 | 0.003489 | 0.020280 |
| 228 | -1.081038 | -309.828767 | 49.311080 | 49.310780 | 0.003489 | 0.020280 |
| 229 | -1.082433 | 310.602445 | 49.434215 | 49.433914 | 0.003485 | 0.020229 |
| 230 | -1.082433 | -310.602445 | 49.434215 | 49.433914 | 0.003485 | 0.020229 |
| 231 | -1.084088 | 322.662762 | 51.353663 | 51.353373 | 0.003360 | 0.019473 |
| 232 | -1.084088 | -322.662762 | 51.353663 | 51.353373 | 0.003360 | 0.019473 |
| 233 | -1.084503 | -322.669036 | 51.354662 | 51.354372 | 0.003361 | 0.019473 |
| 234 | -1.084503 | 322.669036 | 51.354662 | 51.354372 | 0.003361 | 0.019473 |
| 235 | -1.085005 | -319.745514 | 50.889372 | 50.889079 | 0.003393 | 0.019651 |
| 236 | -1.085005 | 319.745514 | 50.889372 | 50.889079 | 0.003393 | 0.019651 |
| 237 | -1.085145 | 319.750916 | 50.890232 | 50.889939 | 0.003394 | 0.019650 |
| 238 | -1.085145 | -319.750916 | 50.890232 | 50.889939 | 0.003394 | 0.019650 |
| 239 | -1.086265 | -329.094966 | 52.377376 | 52.377091 | 0.003301 | 0.019092 |
| 240 | -1.086265 | 329.094966 | 52.377376 | 52.377091 | 0.003301 | 0.019092 |
| 241 | -1.086372 | -326.932381 | 52.033192 | 52.032904 | 0.003323 | 0.019219 |
| 242 | -1.086372 | 326.932381 | 52.033192 | 52.032904 | 0.003323 | 0.019219 |
| 243 | -1.086417 | 329.063936 | 52.372437 | 52.372152 | 0.003302 | 0.019094 |
| 244 | -1.086417 | -329.063936 | 52.372437 | 52.372152 | 0.003302 | 0.019094 |
| 245 | -1.087051 | 313.245575 | 49.854882 | 49.854582 | 0.003470 | 0.020058 |
| 246 | -1.087051 | -313.245575 | 49.854882 | 49.854582 | 0.003470 | 0.020058 |
| 247 | -1.087414 | 313.457338 | 49.888585 | 49.888285 | 0.003469 | 0.020045 |
| 248 | -1.087414 | -313.457338 | 49.888585 | 49.888285 | 0.003469 | 0.020045 |
| 249 | -1.087499 | 313.508013 | 49.896650 | 49.896350 | 0.003469 | 0.020042 |
| 250 | -1.087499 | -313.508013 | 49.896650 | 49.896350 | 0.003469 | 0.020042 |
| 251 | -1.087543 | 313.538425 | 49.901490 | 49.901190 | 0.003469 | 0.020040 |
| 252 | -1.087543 | -313.538425 | 49.901490 | 49.901190 | 0.003469 | 0.020040 |
| 253 | -1.087735 | 326.918220 | 52.030939 | 52.030651 | 0.003327 | 0.019219 |
| 254 | -1.087735 | -326.918220 | 52.030939 | 52.030651 | 0.003327 | 0.019219 |
| 255 | -1.089451 | 314.692046 | 50.085095 | 50.084795 | 0.003462 | 0.019966 |
| 256 | -1.089451 | -314.692046 | 50.085095 | 50.084795 | 0.003462 | 0.019966 |
| 257 | -1.089795 | -330.845038 | 52.655909 | 52.655623 | 0.003294 | 0.018991 |
| 258 | -1.089795 | 330.845038 | 52.655909 | 52.655623 | 0.003294 | 0.018991 |
| 259 | -1.091434 | -330.806905 | 52.649841 | 52.649554 | 0.003299 | 0.018994 |
| 260 | -1.091434 | 330.806905 | 52.649841 | 52.649554 | 0.003299 | 0.018994 |
| 261 | -1.091577 | 323.712470 | 51.520733 | 51.520440 | 0.003372 | 0.019410 |
| 262 | -1.091577 | -323.712470 | 51.520733 | 51.520440 | 0.003372 | 0.019410 |
| 263 | -1.091720 | -327.088590 | 52.058056 | 52.057766 | 0.003338 | 0.019209 |
| 264 | -1.091720 | 327.088590 | 52.058056 | 52.057766 | 0.003338 | 0.019209 |
| 265 | -1.091742 | -323.846011 | 51.541986 | 51.541693 | 0.003371 | 0.019402 |
| 266 | -1.091742 | 323.846011 | 51.541986 | 51.541693 | 0.003371 | 0.019402 |
| 267 | -1.092874 | 327.210801 | 52.077507 | 52.077216 | 0.003340 | 0.019202 |
| 268 | -1.092874 | -327.210801 | 52.077507 | 52.077216 | 0.003340 | 0.019202 |
| 269 | -1.092906 | -316.871195 | 50.431917 | 50.431617 | 0.003449 | 0.019829 |
| 270 | -1.092906 | 316.871195 | 50.431917 | 50.431617 | 0.003449 | 0.019829 |
| 271 | -1.092933 | 316.892539 | 50.435314 | 50.435014 | 0.003449 | 0.019827 |
| 272 | -1.092933 | -316.892539 | 50.435314 | 50.435014 | 0.003449 | 0.019827 |
| 273 | -1.092962 | 316.907185 | 50.437645 | 50.437345 | 0.003449 | 0.019827 |
| 274 | -1.092962 | -316.907185 | 50.437645 | 50.437345 | 0.003449 | 0.019827 |
| 275 | -1.092985 | -316.926030 | 50.440644 | 50.440344 | 0.003449 | 0.019825 |
| 276 | -1.092985 | 316.926030 | 50.440644 | 50.440344 | 0.003449 | 0.019825 |
| 277 | -1.093283 | -332.332221 | 52.892602 | 52.892316 | 0.003290 | 0.018906 |
| 278 | -1.093283 | 332.332221 | 52.892602 | 52.892316 | 0.003290 | 0.018906 |
| 279 | -1.093458 | 332.343157 | 52.894343 | 52.894056 | 0.003290 | 0.018906 |
| 280 | -1.093458 | -332.343157 | 52.894343 | 52.894056 | 0.003290 | 0.018906 |
| 281 | -1.094656 | 325.174138 | 51.753365 | 51.753071 | 0.003366 | 0.019323 |
| 282 | -1.094656 | -325.174138 | 51.753365 | 51.753071 | 0.003366 | 0.019323 |
| 283 | -1.095311 | 325.124568 | 51.745476 | 51.745182 | 0.003369 | 0.019325 |
| 284 | -1.095311 | -325.124568 | 51.745476 | 51.745182 | 0.003369 | 0.019325 |
| 285 | -1.096829 | -332.066508 | 52.850314 | 52.850026 | 0.003303 | 0.018921 |
| 286 | -1.096829 | 332.066508 | 52.850314 | 52.850026 | 0.003303 | 0.018921 |
| 287 | -1.097490 | -319.989534 | 50.928216 | 50.927916 | 0.003430 | 0.019636 |
| 288 | -1.097490 | 319.989534 | 50.928216 | 50.927916 | 0.003430 | 0.019636 |
| 289 | -1.097493 | 319.994348 | 50.928982 | 50.928682 | 0.003430 | 0.019635 |
| 290 | -1.097493 | -319.994348 | 50.928982 | 50.928682 | 0.003430 | 0.019635 |
| 291 | -1.097519 | -320.011922 | 50.931779 | 50.931479 | 0.003430 | 0.019634 |
| 292 | -1.097519 | 320.011922 | 50.931779 | 50.931479 | 0.003430 | 0.019634 |
| 293 | -1.097543 | -320.026563 | 50.934109 | 50.933809 | 0.003430 | 0.019633 |
| 294 | -1.097543 | 320.026563 | 50.934109 | 50.933809 | 0.003430 | 0.019633 |
| 295 | -1.098669 | -331.385423 | 52.741918 | 52.741628 | 0.003315 | 0.018960 |
| 296 | -1.098669 | 331.385423 | 52.741918 | 52.741628 | 0.003315 | 0.018960 |
| 297 | -1.100859 | -326.436651 | 51.954302 | 51.954007 | 0.003372 | 0.019248 |
| 298 | -1.100859 | 326.436651 | 51.954302 | 51.954007 | 0.003372 | 0.019248 |
| 299 | -1.101053 | -326.436812 | 51.954328 | 51.954032 | 0.003373 | 0.019248 |
| 300 | -1.101053 | 326.436812 | 51.954328 | 51.954032 | 0.003373 | 0.019248 |
| 301 | -1.101292 | 322.822286 | 51.379062 | 51.378763 | 0.003411 | 0.019463 |
| 302 | -1.101292 | -322.822286 | 51.379062 | 51.378763 | 0.003411 | 0.019463 |
| 303 | -1.101293 | 322.823898 | 51.379318 | 51.379019 | 0.003411 | 0.019463 |
| 304 | -1.101293 | -322.823898 | 51.379318 | 51.379019 | 0.003411 | 0.019463 |
| 305 | -1.101309 | 322.834412 | 51.380991 | 51.380692 | 0.003411 | 0.019463 |
| 306 | -1.101309 | -322.834412 | 51.380991 | 51.380692 | 0.003411 | 0.019463 |
| 307 | -1.101331 | 322.849724 | 51.383428 | 51.383129 | 0.003411 | 0.019462 |
| 308 | -1.101331 | -322.849724 | 51.383428 | 51.383129 | 0.003411 | 0.019462 |
| 309 | -1.102161 | -330.407444 | 52.586271 | 52.585978 | 0.003336 | 0.019016 |
| 310 | -1.102161 | 330.407444 | 52.586271 | 52.585978 | 0.003336 | 0.019016 |
| 311 | -1.102206 | -328.348838 | 52.258635 | 52.258341 | 0.003357 | 0.019136 |
| 312 | -1.102206 | 328.348838 | 52.258635 | 52.258341 | 0.003357 | 0.019136 |
| 313 | -1.102281 | -328.322944 | 52.254514 | 52.254220 | 0.003357 | 0.019137 |
| 314 | -1.102281 | 328.322944 | 52.254514 | 52.254220 | 0.003357 | 0.019137 |
| 315 | -1.103460 | 330.498141 | 52.600706 | 52.600413 | 0.003339 | 0.019011 |
| 316 | -1.103460 | -330.498141 | 52.600706 | 52.600413 | 0.003339 | 0.019011 |
| 317 | -1.104404 | -325.357719 | 51.782588 | 51.782289 | 0.003394 | 0.019312 |
| 318 | -1.104404 | 325.357719 | 51.782588 | 51.782289 | 0.003394 | 0.019312 |
| 319 | -1.104408 | -325.362658 | 51.783374 | 51.783075 | 0.003394 | 0.019311 |
| 320 | -1.104408 | 325.362658 | 51.783374 | 51.783075 | 0.003394 | 0.019311 |
| 321 | -1.104432 | 325.380976 | 51.786289 | 51.785991 | 0.003394 | 0.019310 |
| 322 | -1.104432 | -325.380976 | 51.786289 | 51.785991 | 0.003394 | 0.019310 |
| 323 | -1.104447 | -325.392512 | 51.788125 | 51.787827 | 0.003394 | 0.019310 |
| 324 | -1.104447 | 325.392512 | 51.788125 | 51.787827 | 0.003394 | 0.019310 |
| 325 | -1.104643 | 329.605095 | 52.458575 | 52.458280 | 0.003351 | 0.019063 |
| 326 | -1.104643 | -329.605095 | 52.458575 | 52.458280 | 0.003351 | 0.019063 |
| 327 | -1.104721 | 329.588858 | 52.455991 | 52.455696 | 0.003352 | 0.019064 |
| 328 | -1.104721 | -329.588858 | 52.455991 | 52.455696 | 0.003352 | 0.019064 |
| 329 | -1.106744 | -327.432658 | 52.112824 | 52.112526 | 0.003380 | 0.019189 |
| 330 | -1.106744 | 327.432658 | 52.112824 | 52.112526 | 0.003380 | 0.019189 |
| 331 | -1.106932 | 327.609429 | 52.140958 | 52.140660 | 0.003379 | 0.019179 |
| 332 | -1.106932 | -327.609429 | 52.140958 | 52.140660 | 0.003379 | 0.019179 |
| 333 | -1.106956 | -327.628565 | 52.144003 | 52.143706 | 0.003379 | 0.019178 |
| 334 | -1.106956 | 327.628565 | 52.144003 | 52.143706 | 0.003379 | 0.019178 |
| 335 | -1.106973 | 327.644199 | 52.146491 | 52.146194 | 0.003379 | 0.019177 |
| 336 | -1.106973 | -327.644199 | 52.146491 | 52.146194 | 0.003379 | 0.019177 |
| 337 | -1.107211 | -327.869981 | 52.182426 | 52.182128 | 0.003377 | 0.019164 |
| 338 | -1.107211 | 327.869981 | 52.182426 | 52.182128 | 0.003377 | 0.019164 |
| 339 | -1.107565 | 331.532240 | 52.765289 | 52.764995 | 0.003341 | 0.018952 |
| 340 | -1.107565 | -331.532240 | 52.765289 | 52.764995 | 0.003341 | 0.018952 |
| 341 | -1.107916 | -333.461804 | 53.072387 | 53.072094 | 0.003322 | 0.018842 |
| 342 | -1.107916 | 333.461804 | 53.072387 | 53.072094 | 0.003322 | 0.018842 |
| 343 | -1.108211 | 331.513313 | 52.762277 | 52.761983 | 0.003343 | 0.018953 |
| 344 | -1.108211 | -331.513313 | 52.762277 | 52.761983 | 0.003343 | 0.018953 |
| 345 | -1.108216 | -333.455247 | 53.071344 | 53.071051 | 0.003323 | 0.018843 |
| 346 | -1.108216 | 333.455247 | 53.071344 | 53.071051 | 0.003323 | 0.018843 |
| 347 | -1.108440 | 329.055252 | 52.371067 | 52.370770 | 0.003369 | 0.019095 |
| 348 | -1.108440 | -329.055252 | 52.371067 | 52.370770 | 0.003369 | 0.019095 |
| 349 | -1.108951 | 329.564068 | 52.452047 | 52.451750 | 0.003365 | 0.019065 |
| 350 | -1.108951 | -329.564068 | 52.452047 | 52.451750 | 0.003365 | 0.019065 |
| 351 | -1.108969 | 329.582680 | 52.455010 | 52.454713 | 0.003365 | 0.019064 |
| 352 | -1.108969 | -329.582680 | 52.455010 | 52.454713 | 0.003365 | 0.019064 |
| 353 | -1.108985 | 329.604673 | 52.458510 | 52.458213 | 0.003365 | 0.019063 |
| 354 | -1.108985 | -329.604673 | 52.458510 | 52.458213 | 0.003365 | 0.019063 |
| 355 | -1.109008 | 329.624636 | 52.461687 | 52.461390 | 0.003364 | 0.019062 |
| 356 | -1.109008 | -329.624636 | 52.461687 | 52.461390 | 0.003364 | 0.019062 |
| 357 | -1.109656 | -334.561471 | 53.247405 | 53.247112 | 0.003317 | 0.018780 |
| 358 | -1.109656 | 334.561471 | 53.247405 | 53.247112 | 0.003317 | 0.018780 |
| 359 | -1.109672 | -334.545407 | 53.244848 | 53.244555 | 0.003317 | 0.018781 |
| 360 | -1.109672 | 334.545407 | 53.244848 | 53.244555 | 0.003317 | 0.018781 |
| 361 | -1.110193 | -333.282365 | 53.043830 | 53.043536 | 0.003331 | 0.018852 |
| 362 | -1.110193 | 333.282365 | 53.043830 | 53.043536 | 0.003331 | 0.018852 |
| 363 | -1.110333 | -331.008434 | 52.681925 | 52.681628 | 0.003354 | 0.018982 |
| 364 | -1.110333 | 331.008434 | 52.681925 | 52.681628 | 0.003354 | 0.018982 |
| 365 | -1.110341 | 331.018034 | 52.683453 | 52.683156 | 0.003354 | 0.018981 |
| 366 | -1.110341 | -331.018034 | 52.683453 | 52.683156 | 0.003354 | 0.018981 |
| 367 | -1.110385 | -333.294673 | 53.045789 | 53.045495 | 0.003332 | 0.018852 |
| 368 | -1.110385 | 333.294673 | 53.045789 | 53.045495 | 0.003332 | 0.018852 |
| 369 | -1.110518 | 331.208602 | 52.713783 | 52.713486 | 0.003353 | 0.018970 |
| 370 | -1.110518 | -331.208602 | 52.713783 | 52.713486 | 0.003353 | 0.018970 |
| 371 | -1.110520 | 331.214085 | 52.714655 | 52.714359 | 0.003353 | 0.018970 |
| 372 | -1.110520 | -331.214085 | 52.714655 | 52.714359 | 0.003353 | 0.018970 |
| 373 | -1.110649 | 331.351732 | 52.736562 | 52.736266 | 0.003352 | 0.018962 |
| 374 | -1.110649 | -331.351732 | 52.736562 | 52.736266 | 0.003352 | 0.018962 |
| 375 | -1.110652 | -331.363928 | 52.738503 | 52.738207 | 0.003352 | 0.018962 |
| 376 | -1.110652 | 331.363928 | 52.738503 | 52.738207 | 0.003352 | 0.018962 |
| 377 | -1.111317 | -332.101213 | 52.855846 | 52.855550 | 0.003346 | 0.018919 |
| 378 | -1.111317 | 332.101213 | 52.855846 | 52.855550 | 0.003346 | 0.018919 |
| 379 | -1.111358 | -332.145988 | 52.862972 | 52.862676 | 0.003346 | 0.018917 |
| 380 | -1.111358 | 332.145988 | 52.862972 | 52.862676 | 0.003346 | 0.018917 |
| 381 | -1.111730 | -332.575059 | 52.931260 | 52.930965 | 0.003343 | 0.018893 |
| 382 | -1.111730 | 332.575059 | 52.931260 | 52.930965 | 0.003343 | 0.018893 |
| 383 | -1.111730 | 332.577756 | 52.931690 | 52.931394 | 0.003343 | 0.018892 |
| 384 | -1.111730 | -332.577756 | 52.931690 | 52.931394 | 0.003343 | 0.018892 |
| 385 | -1.111733 | -332.582902 | 52.932509 | 52.932213 | 0.003343 | 0.018892 |
| 386 | -1.111733 | 332.582902 | 52.932509 | 52.932213 | 0.003343 | 0.018892 |
| 387 | -1.111733 | 332.581750 | 52.932325 | 52.932029 | 0.003343 | 0.018892 |
| 388 | -1.111733 | -332.581750 | 52.932325 | 52.932029 | 0.003343 | 0.018892 |
| 389 | -1.111895 | -335.183640 | 53.346427 | 53.346133 | 0.003317 | 0.018746 |
| 390 | -1.111895 | 335.183640 | 53.346427 | 53.346133 | 0.003317 | 0.018746 |
| 391 | -1.111918 | -335.185684 | 53.346752 | 53.346459 | 0.003317 | 0.018745 |
| 392 | -1.111918 | 335.185684 | 53.346752 | 53.346459 | 0.003317 | 0.018745 |
| 393 | -1.111977 | -337.042524 | 53.642276 | 53.641984 | 0.003299 | 0.018642 |
| 394 | -1.111977 | 337.042524 | 53.642276 | 53.641984 | 0.003299 | 0.018642 |
| 395 | -1.111984 | 337.038066 | 53.641566 | 53.641274 | 0.003299 | 0.018642 |
| 396 | -1.111984 | -337.038066 | 53.641566 | 53.641274 | 0.003299 | 0.018642 |
| 397 | -1.113433 | 336.128317 | 53.496777 | 53.496483 | 0.003313 | 0.018693 |
| 398 | -1.113433 | -336.128317 | 53.496777 | 53.496483 | 0.003313 | 0.018693 |
| 399 | -1.113437 | 336.131297 | 53.497251 | 53.496957 | 0.003312 | 0.018693 |
| 400 | -1.113437 | -336.131297 | 53.497251 | 53.496957 | 0.003312 | 0.018693 |
| 401 | -1.113648 | -338.105396 | 53.811437 | 53.811145 | 0.003294 | 0.018584 |
| 402 | -1.113648 | 338.105396 | 53.811437 | 53.811145 | 0.003294 | 0.018584 |
| 403 | -1.113653 | -338.102774 | 53.811020 | 53.810728 | 0.003294 | 0.018584 |
| 404 | -1.113653 | 338.102774 | 53.811020 | 53.810728 | 0.003294 | 0.018584 |
| 405 | -1.113881 | 335.273046 | 53.360657 | 53.360363 | 0.003322 | 0.018741 |
| 406 | -1.113881 | -335.273046 | 53.360657 | 53.360363 | 0.003322 | 0.018741 |
| 407 | -1.114271 | 335.810669 | 53.446222 | 53.445928 | 0.003318 | 0.018710 |
| 408 | -1.114271 | -335.810669 | 53.446222 | 53.445928 | 0.003318 | 0.018710 |
| 409 | -1.114682 | -337.420697 | 53.702465 | 53.702172 | 0.003304 | 0.018621 |
| 410 | -1.114682 | 337.420697 | 53.702465 | 53.702172 | 0.003304 | 0.018621 |
| 411 | -1.114685 | -337.421129 | 53.702534 | 53.702241 | 0.003304 | 0.018621 |
| 412 | -1.114685 | 337.421129 | 53.702534 | 53.702241 | 0.003304 | 0.018621 |
| 413 | -1.114745 | -339.534726 | 54.038921 | 54.038630 | 0.003283 | 0.018505 |
| 414 | -1.114745 | 339.534726 | 54.038921 | 54.038630 | 0.003283 | 0.018505 |
| 415 | -1.114754 | 339.527234 | 54.037729 | 54.037438 | 0.003283 | 0.018506 |
| 416 | -1.114754 | -339.527234 | 54.037729 | 54.037438 | 0.003283 | 0.018506 |
| 417 | -1.115312 | 74.207184 | 11.811774 | 11.810440 | 0.015028 | 0.084671 |
| 418 | -1.115312 | -74.207184 | 11.811774 | 11.810440 | 0.015028 | 0.084671 |
| 419 | -1.115588 | 338.574851 | 53.886154 | 53.885861 | 0.003295 | 0.018558 |
| 420 | -1.115588 | -338.574851 | 53.886154 | 53.885861 | 0.003295 | 0.018558 |
| 421 | -1.115590 | 338.573805 | 53.885987 | 53.885695 | 0.003295 | 0.018558 |
| 422 | -1.115590 | -338.573805 | 53.885987 | 53.885695 | 0.003295 | 0.018558 |
| 423 | -1.115783 | -340.920059 | 54.259403 | 54.259113 | 0.003273 | 0.018430 |
| 424 | -1.115783 | 340.920059 | 54.259403 | 54.259113 | 0.003273 | 0.018430 |
| 425 | -1.115870 | 341.161924 | 54.297897 | 54.297607 | 0.003271 | 0.018417 |
| 426 | -1.115870 | -341.161924 | 54.297897 | 54.297607 | 0.003271 | 0.018417 |
| 427 | -1.115916 | 340.879701 | 54.252980 | 54.252689 | 0.003274 | 0.018432 |
| 428 | -1.115916 | -340.879701 | 54.252980 | 54.252689 | 0.003274 | 0.018432 |
| 429 | -1.116022 | -341.105628 | 54.288937 | 54.288647 | 0.003272 | 0.018420 |
| 430 | -1.116022 | 341.105628 | 54.288937 | 54.288647 | 0.003272 | 0.018420 |
| 431 | -1.116122 | 339.695422 | 54.064497 | 54.064206 | 0.003286 | 0.018497 |
| 432 | -1.116122 | -339.695422 | 54.064497 | 54.064206 | 0.003286 | 0.018497 |
| 433 | -1.116123 | 339.694299 | 54.064319 | 54.064027 | 0.003286 | 0.018497 |
| 434 | -1.116123 | -339.694299 | 54.064319 | 54.064027 | 0.003286 | 0.018497 |
| 435 | -1.116483 | -339.281941 | 53.998690 | 53.998398 | 0.003291 | 0.018519 |
| 436 | -1.116483 | 339.281941 | 53.998690 | 53.998398 | 0.003291 | 0.018519 |
| 437 | -1.116592 | -340.579316 | 54.205173 | 54.204882 | 0.003278 | 0.018449 |
| 438 | -1.116592 | 340.579316 | 54.205173 | 54.204882 | 0.003278 | 0.018449 |
| 439 | -1.116631 | -339.550217 | 54.041388 | 54.041095 | 0.003289 | 0.018504 |
| 440 | -1.116631 | 339.550217 | 54.041388 | 54.041095 | 0.003289 | 0.018504 |
| 441 | -1.116657 | -340.591235 | 54.207070 | 54.206779 | 0.003279 | 0.018448 |
| 442 | -1.116657 | 340.591235 | 54.207070 | 54.206779 | 0.003279 | 0.018448 |
| 443 | -1.117075 | 342.131792 | 54.452256 | 54.451966 | 0.003265 | 0.018365 |
| 444 | -1.117075 | -342.131792 | 54.452256 | 54.451966 | 0.003265 | 0.018365 |
| 445 | -1.117094 | 342.130820 | 54.452101 | 54.451811 | 0.003265 | 0.018365 |
| 446 | -1.117094 | -342.130820 | 54.452101 | 54.451811 | 0.003265 | 0.018365 |
| 447 | -1.117293 | -341.489178 | 54.349982 | 54.349691 | 0.003272 | 0.018399 |
| 448 | -1.117293 | 341.489178 | 54.349982 | 54.349691 | 0.003272 | 0.018399 |
| 449 | -1.117322 | 341.487729 | 54.349751 | 54.349460 | 0.003272 | 0.018399 |
| 450 | -1.117322 | -341.487729 | 54.349751 | 54.349460 | 0.003272 | 0.018399 |
| 451 | -1.117513 | -342.198690 | 54.462903 | 54.462613 | 0.003266 | 0.018361 |
| 452 | -1.117513 | 342.198690 | 54.462903 | 54.462613 | 0.003266 | 0.018361 |
| 453 | -1.117532 | -342.200560 | 54.463201 | 54.462911 | 0.003266 | 0.018361 |
| 454 | -1.117532 | 342.200560 | 54.463201 | 54.462911 | 0.003266 | 0.018361 |
| 455 | -1.117646 | 343.037593 | 54.596418 | 54.596129 | 0.003258 | 0.018316 |
| 456 | -1.117646 | -343.037593 | 54.596418 | 54.596129 | 0.003258 | 0.018316 |
| 457 | -1.117646 | -343.037592 | 54.596418 | 54.596128 | 0.003258 | 0.018316 |
| 458 | -1.117646 | 343.037592 | 54.596418 | 54.596128 | 0.003258 | 0.018316 |
| 459 | -1.117777 | -341.853412 | 54.407951 | 54.407660 | 0.003270 | 0.018380 |
| 460 | -1.117777 | 341.853412 | 54.407951 | 54.407660 | 0.003270 | 0.018380 |
| 461 | -1.117846 | -342.009266 | 54.432756 | 54.432465 | 0.003268 | 0.018371 |
| 462 | -1.117846 | 342.009266 | 54.432756 | 54.432465 | 0.003268 | 0.018371 |
| 463 | -1.118011 | -342.479554 | 54.507604 | 54.507314 | 0.003264 | 0.018346 |
| 464 | -1.118011 | 342.479554 | 54.507604 | 54.507314 | 0.003264 | 0.018346 |
| 465 | -1.118029 | -342.507545 | 54.512059 | 54.511769 | 0.003264 | 0.018345 |
| 466 | -1.118029 | 342.507545 | 54.512059 | 54.511769 | 0.003264 | 0.018345 |
| 467 | -1.118087 | -343.710417 | 54.703501 | 54.703212 | 0.003253 | 0.018280 |
| 468 | -1.118087 | 343.710417 | 54.703501 | 54.703212 | 0.003253 | 0.018280 |
| 469 | -1.118087 | 343.710417 | 54.703501 | 54.703212 | 0.003253 | 0.018280 |
| 470 | -1.118087 | -343.710417 | 54.703501 | 54.703212 | 0.003253 | 0.018280 |
| 471 | -1.118088 | -342.821813 | 54.562076 | 54.561786 | 0.003261 | 0.018328 |
| 472 | -1.118088 | 342.821813 | 54.562076 | 54.561786 | 0.003261 | 0.018328 |
| 473 | -1.118088 | 342.822024 | 54.562110 | 54.561820 | 0.003261 | 0.018328 |
| 474 | -1.118088 | -342.822024 | 54.562110 | 54.561820 | 0.003261 | 0.018328 |
| 475 | -1.118409 | -344.494693 | 54.828322 | 54.828033 | 0.003247 | 0.018239 |
| 476 | -1.118409 | 344.494693 | 54.828322 | 54.828033 | 0.003247 | 0.018239 |
| 477 | -1.118409 | -344.494693 | 54.828322 | 54.828033 | 0.003247 | 0.018239 |
| 478 | -1.118409 | 344.494693 | 54.828322 | 54.828033 | 0.003247 | 0.018239 |
| 479 | -1.118504 | -343.644764 | 54.693053 | 54.692763 | 0.003255 | 0.018284 |
| 480 | -1.118504 | 343.644764 | 54.693053 | 54.692763 | 0.003255 | 0.018284 |
| 481 | -1.118539 | -343.740135 | 54.708231 | 54.707942 | 0.003254 | 0.018279 |
| 482 | -1.118539 | 343.740135 | 54.708231 | 54.707942 | 0.003254 | 0.018279 |
| 483 | -1.118797 | 345.352018 | 54.964769 | 54.964481 | 0.003240 | 0.018194 |
| 484 | -1.118797 | -345.352018 | 54.964769 | 54.964481 | 0.003240 | 0.018194 |
| 485 | -1.118797 | 345.352018 | 54.964769 | 54.964481 | 0.003240 | 0.018194 |
| 486 | -1.118797 | -345.352018 | 54.964769 | 54.964481 | 0.003240 | 0.018194 |
| 487 | -1.118942 | -344.942716 | 54.899627 | 54.899338 | 0.003244 | 0.018215 |
| 488 | -1.118942 | 344.942716 | 54.899627 | 54.899338 | 0.003244 | 0.018215 |
| 489 | -1.118960 | -345.001926 | 54.909051 | 54.908762 | 0.003243 | 0.018212 |
| 490 | -1.118960 | 345.001926 | 54.909051 | 54.908762 | 0.003243 | 0.018212 |
| 491 | -1.119142 | 346.233999 | 55.105140 | 55.104852 | 0.003232 | 0.018147 |
| 492 | -1.119142 | -346.233999 | 55.105140 | 55.104852 | 0.003232 | 0.018147 |
| 493 | -1.119142 | 346.233999 | 55.105140 | 55.104852 | 0.003232 | 0.018147 |
| 494 | -1.119142 | -346.233999 | 55.105140 | 55.104852 | 0.003232 | 0.018147 |
| 495 | -1.119223 | 345.921418 | 55.055392 | 55.055104 | 0.003235 | 0.018164 |
| 496 | -1.119223 | -345.921418 | 55.055392 | 55.055104 | 0.003235 | 0.018164 |
| 497 | -1.119232 | 345.957711 | 55.061168 | 55.060880 | 0.003235 | 0.018162 |
| 498 | -1.119232 | -345.957711 | 55.061168 | 55.060880 | 0.003235 | 0.018162 |
| 499 | -1.119414 | -346.692126 | 55.178053 | 55.177766 | 0.003229 | 0.018123 |
| 500 | -1.119414 | 346.692126 | 55.178053 | 55.177766 | 0.003229 | 0.018123 |
| 501 | -1.119419 | -346.714128 | 55.181555 | 55.181267 | 0.003229 | 0.018122 |
| 502 | -1.119419 | 346.714128 | 55.181555 | 55.181267 | 0.003229 | 0.018122 |
| 503 | -1.119436 | -347.160911 | 55.252662 | 55.252375 | 0.003225 | 0.018099 |
| 504 | -1.119436 | 347.160911 | 55.252662 | 55.252375 | 0.003225 | 0.018099 |
| 505 | -1.119436 | -347.160911 | 55.252662 | 55.252375 | 0.003225 | 0.018099 |
| 506 | -1.119436 | 347.160911 | 55.252662 | 55.252375 | 0.003225 | 0.018099 |
| 507 | -1.119550 | -347.324058 | 55.278628 | 55.278341 | 0.003223 | 0.018090 |
| 508 | -1.119550 | 347.324058 | 55.278628 | 55.278341 | 0.003223 | 0.018090 |
| 509 | -1.119554 | -347.339241 | 55.281044 | 55.280757 | 0.003223 | 0.018089 |
| 510 | -1.119554 | 347.339241 | 55.281044 | 55.280757 | 0.003223 | 0.018089 |
| 511 | -1.119645 | 347.818682 | 55.357349 | 55.357063 | 0.003219 | 0.018065 |
| 512 | -1.119645 | -347.818682 | 55.357349 | 55.357063 | 0.003219 | 0.018065 |
| 513 | -1.119648 | 347.835778 | 55.360070 | 55.359783 | 0.003219 | 0.018064 |
| 514 | -1.119648 | -347.835778 | 55.360070 | 55.359783 | 0.003219 | 0.018064 |
| 515 | -1.119700 | 348.136761 | 55.407973 | 55.407686 | 0.003216 | 0.018048 |
| 516 | -1.119700 | -348.136761 | 55.407973 | 55.407686 | 0.003216 | 0.018048 |
| 517 | -1.119702 | 348.145161 | 55.409310 | 55.409023 | 0.003216 | 0.018048 |
| 518 | -1.119702 | -348.145161 | 55.409310 | 55.409023 | 0.003216 | 0.018048 |
| 519 | -1.119747 | 348.428886 | 55.454466 | 55.454180 | 0.003214 | 0.018033 |
| 520 | -1.119747 | -348.428886 | 55.454466 | 55.454180 | 0.003214 | 0.018033 |
| 521 | -1.119747 | 348.429776 | 55.454608 | 55.454321 | 0.003214 | 0.018033 |
| 522 | -1.119747 | -348.429776 | 55.454608 | 55.454321 | 0.003214 | 0.018033 |
| 523 | -1.119798 | -348.784816 | 55.511114 | 55.510828 | 0.003211 | 0.018015 |
| 524 | -1.119798 | 348.784816 | 55.511114 | 55.510828 | 0.003211 | 0.018015 |
| 525 | -1.119799 | 348.788549 | 55.511708 | 55.511422 | 0.003211 | 0.018014 |
| 526 | -1.119799 | -348.788549 | 55.511708 | 55.511422 | 0.003211 | 0.018014 |
| 527 | -1.119838 | 349.087485 | 55.559285 | 55.558999 | 0.003208 | 0.017999 |
| 528 | -1.119838 | -349.087485 | 55.559285 | 55.558999 | 0.003208 | 0.017999 |
| 529 | -1.119838 | 349.088940 | 55.559516 | 55.559230 | 0.003208 | 0.017999 |
| 530 | -1.119838 | -349.088940 | 55.559516 | 55.559230 | 0.003208 | 0.017999 |
| 531 | -1.119864 | 349.305750 | 55.594023 | 55.593737 | 0.003206 | 0.017988 |
| 532 | -1.119864 | -349.305750 | 55.594023 | 55.593737 | 0.003206 | 0.017988 |
| 533 | -1.119864 | 349.306973 | 55.594217 | 55.593931 | 0.003206 | 0.017988 |
| 534 | -1.119864 | -349.306973 | 55.594217 | 55.593931 | 0.003206 | 0.017988 |
| 535 | -1.119888 | -349.526188 | 55.629106 | 55.628821 | 0.003204 | 0.017976 |
| 536 | -1.119888 | 349.526188 | 55.629106 | 55.628821 | 0.003204 | 0.017976 |
| 537 | -1.119888 | -349.527157 | 55.629260 | 55.628975 | 0.003204 | 0.017976 |
| 538 | -1.119888 | 349.527157 | 55.629260 | 55.628975 | 0.003204 | 0.017976 |
| 539 | -1.119908 | -349.726171 | 55.660934 | 55.660649 | 0.003202 | 0.017966 |
| 540 | -1.119908 | 349.726171 | 55.660934 | 55.660649 | 0.003202 | 0.017966 |
| 541 | -1.119908 | -349.726960 | 55.661060 | 55.660774 | 0.003202 | 0.017966 |
| 542 | -1.119908 | 349.726960 | 55.661060 | 55.660774 | 0.003202 | 0.017966 |
| 543 | -1.119924 | 349.905629 | 55.689496 | 55.689211 | 0.003201 | 0.017957 |
| 544 | -1.119924 | -349.905629 | 55.689496 | 55.689211 | 0.003201 | 0.017957 |
| 545 | -1.119924 | -349.906343 | 55.689609 | 55.689324 | 0.003201 | 0.017957 |
| 546 | -1.119924 | 349.906343 | 55.689609 | 55.689324 | 0.003201 | 0.017957 |
| 547 | -1.119938 | 350.066095 | 55.715034 | 55.714749 | 0.003199 | 0.017949 |
| 548 | -1.119938 | -350.066095 | 55.715034 | 55.714749 | 0.003199 | 0.017949 |
| 549 | -1.119938 | 350.067052 | 55.715187 | 55.714902 | 0.003199 | 0.017949 |
| 550 | -1.119938 | -350.067052 | 55.715187 | 55.714902 | 0.003199 | 0.017949 |
| 551 | -1.119946 | -350.175587 | 55.732461 | 55.732176 | 0.003198 | 0.017943 |
| 552 | -1.119946 | 350.175587 | 55.732461 | 55.732176 | 0.003198 | 0.017943 |
| 553 | -1.119947 | 350.185229 | 55.733995 | 55.733710 | 0.003198 | 0.017942 |
| 554 | -1.119947 | -350.185229 | 55.733995 | 55.733710 | 0.003198 | 0.017942 |
| 555 | -1.119950 | -350.224672 | 55.740273 | 55.739988 | 0.003198 | 0.017940 |
| 556 | -1.119950 | 350.224672 | 55.740273 | 55.739988 | 0.003198 | 0.017940 |
| 557 | -1.119950 | -350.225655 | 55.740429 | 55.740144 | 0.003198 | 0.017940 |
| 558 | -1.119950 | 350.225655 | 55.740429 | 55.740144 | 0.003198 | 0.017940 |
| 559 | -1.119959 | -350.360870 | 55.761949 | 55.761664 | 0.003197 | 0.017933 |
| 560 | -1.119959 | 350.360870 | 55.761949 | 55.761664 | 0.003197 | 0.017933 |
| 561 | -1.119959 | -350.360874 | 55.761950 | 55.761665 | 0.003197 | 0.017933 |
| 562 | -1.119959 | 350.360874 | 55.761950 | 55.761665 | 0.003197 | 0.017933 |
| 563 | -1.119968 | -350.498253 | 55.783814 | 55.783530 | 0.003195 | 0.017926 |
| 564 | -1.119968 | 350.498253 | 55.783814 | 55.783530 | 0.003195 | 0.017926 |
| 565 | -1.119968 | -350.498253 | 55.783814 | 55.783530 | 0.003195 | 0.017926 |
| 566 | -1.119968 | 350.498253 | 55.783814 | 55.783530 | 0.003195 | 0.017926 |
| 567 | -1.202238 | 215.725167 | 34.334260 | 34.333727 | 0.005573 | 0.029126 |
| 568 | -1.202238 | -215.725167 | 34.334260 | 34.333727 | 0.005573 | 0.029126 |
| 569 | -1.211213 | 218.125851 | 34.716343 | 34.715807 | 0.005553 | 0.028805 |
| 570 | -1.211213 | -218.125851 | 34.716343 | 34.715807 | 0.005553 | 0.028805 |
| 571 | -1.314901 | 27.808792 | 4.430852 | 4.425907 | 0.047231 | 0.225942 |
| 572 | -1.314901 | -27.808792 | 4.430852 | 4.425907 | 0.047231 | 0.225942 |
| 573 | -1.331926 | -193.853508 | 30.853472 | 30.852744 | 0.006871 | 0.032412 |
| 574 | -1.331926 | 193.853508 | 30.853472 | 30.852744 | 0.006871 | 0.032412 |
| 575 | -1.387904 | -100.607607 | 16.013722 | 16.012198 | 0.013794 | 0.062452 |
| 576 | -1.387904 | 100.607607 | 16.013722 | 16.012198 | 0.013794 | 0.062452 |
| 577 | -1.429024 | 181.697099 | 28.918886 | 28.917992 | 0.007865 | 0.034581 |
| 578 | -1.429024 | -181.697099 | 28.918886 | 28.917992 | 0.007865 | 0.034581 |
| 579 | -1.467702 | -29.975101 | 4.776401 | 4.770685 | 0.048905 | 0.209613 |
| 580 | -1.467702 | 29.975101 | 4.776401 | 4.770685 | 0.048905 | 0.209613 |
| 581 | -1.475946 | -189.568142 | 30.171621 | 30.170707 | 0.007786 | 0.033145 |
| 582 | -1.475946 | 189.568142 | 30.171621 | 30.170707 | 0.007786 | 0.033145 |
| 583 | -1.552107 | 121.192652 | 19.289991 | 19.288410 | 0.012806 | 0.051845 |
| 584 | -1.552107 | -121.192652 | 19.289991 | 19.288410 | 0.012806 | 0.051845 |
| 585 | -1.569138 | 147.912741 | 23.542368 | 23.541044 | 0.010608 | 0.042479 |
| 586 | -1.569138 | -147.912741 | 23.542368 | 23.541044 | 0.010608 | 0.042479 |
| 587 | -1.679571 | -166.822478 | 26.551968 | 26.550622 | 0.010068 | 0.037664 |
| 588 | -1.679571 | 166.822478 | 26.551968 | 26.550622 | 0.010068 | 0.037664 |
| 589 | -1.900781 | 146.675612 | 23.346109 | 23.344149 | 0.012958 | 0.042837 |
| 590 | -1.900781 | -146.675612 | 23.346109 | 23.344149 | 0.012958 | 0.042837 |
| 591 | -3.829164 | 6.037090 | 1.137807 | 0.960833 | 0.535618 | 1.040764 |
| 592 | -3.829164 | -6.037090 | 1.137807 | 0.960833 | 0.535618 | 1.040764 |
| 593 | -10.569038 | -2.319301 | 1.722140 | 0.369128 | 0.976758 | 2.709085 |
| 594 | -10.569038 | 2.319301 | 1.722140 | 0.369128 | 0.976758 | 2.709085 |
| 595 | -15.876819 | 0.420548 | 2.527760 | 0.066932 | 0.999649 | 14.940485 |
| 596 | -15.876819 | -0.420548 | 2.527760 | 0.066932 | 0.999649 | 14.940485 |
| 597 | -22.629853 | 0.000000 | 3.601653 | 0.000000 | 1.000000 | inf |
| 598 | -26.267735 | 0.000000 | 4.180640 | 0.000000 | 1.000000 | inf |
| 599 | -29.470709 | 0.000000 | 4.690409 | 0.000000 | 1.000000 | inf |
| 600 | -30.882640 | -35.322709 | 7.467456 | 5.621784 | 0.658206 | 0.177879 |
| 601 | -30.882640 | 35.322709 | 7.467456 | 5.621784 | 0.658206 | 0.177879 |
| 602 | -32.639619 | 0.000000 | 5.194757 | 0.000000 | 1.000000 | inf |
| 603 | -34.172137 | 34.458728 | 7.723753 | 5.484277 | 0.704148 | 0.182339 |
| 604 | -34.172137 | -34.458728 | 7.723753 | 5.484277 | 0.704148 | 0.182339 |
| 605 | -35.603269 | 0.000000 | 5.666436 | 0.000000 | 1.000000 | inf |
| 606 | -36.439642 | -71.349091 | 12.750825 | 11.355561 | 0.454837 | 0.088063 |
| 607 | -36.439642 | 71.349091 | 12.750825 | 11.355561 | 0.454837 | 0.088063 |
| 608 | -37.441913 | 34.065477 | 8.056375 | 5.421689 | 0.739671 | 0.184444 |
| 609 | -37.441913 | -34.065477 | 8.056375 | 5.421689 | 0.739671 | 0.184444 |
| 610 | -38.677028 | 0.000000 | 6.155640 | 0.000000 | 1.000000 | inf |
| 611 | -39.517763 | 106.863529 | 18.133516 | 17.007859 | 0.346841 | 0.058796 |
| 612 | -39.517763 | -106.863529 | 18.133516 | 17.007859 | 0.346841 | 0.058796 |
| 613 | -40.027452 | 70.647916 | 12.923269 | 11.243965 | 0.492953 | 0.088937 |
| 614 | -40.027452 | -70.647916 | 12.923269 | 11.243965 | 0.492953 | 0.088937 |
| 615 | -40.915687 | -33.727014 | 8.439122 | 5.367821 | 0.771636 | 0.186295 |
| 616 | -40.915687 | 33.727014 | 8.439122 | 5.367821 | 0.771636 | 0.186295 |
| 617 | -41.445961 | -142.148833 | 23.565714 | 22.623689 | 0.279912 | 0.044201 |
| 618 | -41.445961 | 142.148833 | 23.565714 | 22.623689 | 0.279912 | 0.044201 |
| 619 | -41.867200 | 0.000000 | 6.663372 | 0.000000 | 1.000000 | inf |
| 620 | -42.728119 | -177.201966 | 29.010864 | 28.202569 | 0.234408 | 0.035458 |
| 621 | -42.728119 | 177.201966 | 29.010864 | 28.202569 | 0.234408 | 0.035458 |
| 622 | -43.280388 | -106.316208 | 18.269108 | 16.920750 | 0.377046 | 0.059099 |
| 623 | -43.280388 | 106.316208 | 18.269108 | 16.920750 | 0.377046 | 0.059099 |
| 624 | -43.336124 | 70.371812 | 13.153375 | 11.200022 | 0.524364 | 0.089286 |
| 625 | -43.336124 | -70.371812 | 13.153375 | 11.200022 | 0.524364 | 0.089286 |
| 626 | -43.703283 | -212.025014 | 34.454227 | 33.744829 | 0.201879 | 0.029634 |
| 627 | -43.703283 | 212.025014 | 34.454227 | 33.744829 | 0.201879 | 0.029634 |
| 628 | -44.464423 | -33.642593 | 8.874096 | 5.354385 | 0.797460 | 0.186763 |
| 629 | -44.464423 | 33.642593 | 8.874096 | 5.354385 | 0.797460 | 0.186763 |
| 630 | -44.565889 | 246.761554 | 39.908680 | 39.273321 | 0.177728 | 0.025463 |
| 631 | -44.565889 | -246.761554 | 39.908680 | 39.273321 | 0.177728 | 0.025463 |
| 632 | -45.337149 | -281.615994 | 45.397682 | 44.820577 | 0.158943 | 0.022311 |
| 633 | -45.337149 | 281.615994 | 45.397682 | 44.820577 | 0.158943 | 0.022311 |
| 634 | -45.383435 | -141.762571 | 23.690192 | 22.562214 | 0.304894 | 0.044322 |
| 635 | -45.383435 | 141.762571 | 23.690192 | 22.562214 | 0.304894 | 0.044322 |
| 636 | -45.635849 | 0.000000 | 7.263171 | 0.000000 | 1.000000 | inf |
| 637 | -45.889180 | 316.668954 | 50.925862 | 50.399429 | 0.143414 | 0.019841 |
| 638 | -45.889180 | -316.668954 | 50.925862 | 50.399429 | 0.143414 | 0.019841 |
| 639 | -46.088938 | 351.858377 | 56.478371 | 56.000000 | 0.129878 | 0.017857 |
| 640 | -46.676008 | 106.038788 | 18.439235 | 16.876597 | 0.402876 | 0.059254 |
| 641 | -46.676008 | -106.038788 | 18.439235 | 16.876597 | 0.402876 | 0.059254 |
| 642 | -46.767036 | 70.167150 | 13.420626 | 11.167449 | 0.554609 | 0.089546 |
| 643 | -46.767036 | -70.167150 | 13.420626 | 11.167449 | 0.554609 | 0.089546 |
| 644 | -46.853578 | -176.902076 | 29.125616 | 28.154840 | 0.256028 | 0.035518 |
| 645 | -46.853578 | 176.902076 | 29.125616 | 28.154840 | 0.256028 | 0.035518 |
| 646 | -47.914776 | 211.777248 | 34.557310 | 33.705396 | 0.220673 | 0.029669 |
| 647 | -47.914776 | -211.777248 | 34.557310 | 33.705396 | 0.220673 | 0.029669 |
| 648 | -48.163221 | 33.591764 | 9.345665 | 5.346295 | 0.820211 | 0.187045 |
| 649 | -48.163221 | -33.591764 | 9.345665 | 5.346295 | 0.820211 | 0.187045 |
| 650 | -48.252213 | 0.000000 | 7.679578 | 0.000000 | 1.000000 | inf |
| 651 | -48.812816 | 246.582271 | 40.006344 | 39.244787 | 0.194189 | 0.025481 |
| 652 | -48.812816 | -246.582271 | 40.006344 | 39.244787 | 0.194189 | 0.025481 |
| 653 | -48.813029 | -141.441198 | 23.813922 | 22.511066 | 0.326231 | 0.044423 |
| 654 | -48.813029 | 141.441198 | 23.813922 | 22.511066 | 0.326231 | 0.044423 |
| 655 | -49.598916 | 281.503089 | 45.492720 | 44.802608 | 0.173520 | 0.022320 |
| 656 | -49.598916 | -281.503089 | 45.492720 | 44.802608 | 0.173520 | 0.022320 |
| 657 | -50.130642 | -105.892724 | 18.646515 | 16.853351 | 0.427884 | 0.059335 |
| 658 | -50.130642 | 105.892724 | 18.646515 | 16.853351 | 0.427884 | 0.059335 |
| 659 | -50.155612 | 316.615486 | 51.019264 | 50.390920 | 0.156461 | 0.019845 |
| 660 | -50.155612 | -316.615486 | 51.019264 | 50.390920 | 0.156461 | 0.019845 |
| 661 | -50.199758 | 70.036291 | 13.714223 | 11.146622 | 0.582573 | 0.089713 |
| 662 | -50.199758 | -70.036291 | 13.714223 | 11.146622 | 0.582573 | 0.089713 |
| 663 | -50.274891 | -176.631667 | 29.228367 | 28.111803 | 0.273758 | 0.035572 |
| 664 | -50.274891 | 176.631667 | 29.228367 | 28.111803 | 0.273758 | 0.035572 |
| 665 | -50.356641 | 351.858377 | 56.570596 | 56.000000 | 0.141673 | 0.017857 |
| 666 | -50.561744 | 0.000000 | 8.047151 | 0.000000 | 1.000000 | inf |
| 667 | -51.384725 | -211.606085 | 34.656889 | 33.678154 | 0.235974 | 0.029693 |
| 668 | -51.384725 | 211.606085 | 34.656889 | 33.678154 | 0.235974 | 0.029693 |
| 669 | -52.064864 | 33.485400 | 9.852221 | 5.329367 | 0.841067 | 0.187640 |
| 670 | -52.064864 | -33.485400 | 9.852221 | 5.329367 | 0.841067 | 0.187640 |
| 671 | -52.270240 | -141.316069 | 23.980382 | 22.491151 | 0.346911 | 0.044462 |
| 672 | -52.270240 | 141.316069 | 23.980382 | 22.491151 | 0.346911 | 0.044462 |
| 673 | -52.308018 | -246.494054 | 40.104345 | 39.230747 | 0.207585 | 0.025490 |
| 674 | -52.308018 | 246.494054 | 40.104345 | 39.230747 | 0.207585 | 0.025490 |
| 675 | -53.091325 | -281.471463 | 45.587508 | 44.797575 | 0.185352 | 0.022323 |
| 676 | -53.091325 | 281.471463 | 45.587508 | 44.797575 | 0.185352 | 0.022323 |
| 677 | -53.523354 | 105.809988 | 18.872114 | 16.840183 | 0.451381 | 0.059382 |
| 678 | -53.523354 | -105.809988 | 18.872114 | 16.840183 | 0.451381 | 0.059382 |
| 679 | -53.632759 | -316.607749 | 51.107559 | 50.389688 | 0.167019 | 0.019845 |
| 680 | -53.632759 | 316.607749 | 51.107559 | 50.389688 | 0.167019 | 0.019845 |
| 681 | -53.713623 | 176.509633 | 29.364326 | 28.092381 | 0.291128 | 0.035597 |
| 682 | -53.713623 | -176.509633 | 29.364326 | 28.092381 | 0.291128 | 0.035597 |
| 683 | -53.826264 | 351.858377 | 56.651466 | 56.000000 | 0.151218 | 0.017857 |
| 684 | -53.844578 | 69.950619 | 14.049269 | 11.132987 | 0.609970 | 0.089823 |
| 685 | -53.844578 | -69.950619 | 14.049269 | 11.132987 | 0.609970 | 0.089823 |
| 686 | -53.932606 | 0.000000 | 8.583641 | 0.000000 | 1.000000 | inf |
| 687 | -54.796128 | 211.491959 | 34.771427 | 33.659991 | 0.250812 | 0.029709 |
| 688 | -54.796128 | -211.491959 | 34.771427 | 33.659991 | 0.250812 | 0.029709 |
| 689 | -55.610859 | 33.325370 | 10.318284 | 5.303897 | 0.857773 | 0.188541 |
| 690 | -55.610859 | -33.325370 | 10.318284 | 5.303897 | 0.857773 | 0.188541 |
| 691 | -55.689855 | 141.250770 | 24.164910 | 22.480758 | 0.366785 | 0.044482 |
| 692 | -55.689855 | -141.250770 | 24.164910 | 22.480758 | 0.366785 | 0.044482 |
| 693 | -55.734550 | -246.399761 | 40.206452 | 39.215740 | 0.220622 | 0.025500 |
| 694 | -55.734550 | 246.399761 | 40.206452 | 39.215740 | 0.220622 | 0.025500 |
| 695 | -56.557649 | 281.398311 | 45.681566 | 44.785932 | 0.197047 | 0.022328 |
| 696 | -56.557649 | -281.398311 | 45.681566 | 44.785932 | 0.197047 | 0.022328 |
| 697 | -57.110723 | -105.753973 | 19.128767 | 16.831268 | 0.475172 | 0.059413 |
| 698 | -57.110723 | 105.753973 | 19.128767 | 16.831268 | 0.475172 | 0.059413 |
| 699 | -57.130384 | 316.567088 | 51.197105 | 50.383217 | 0.177600 | 0.019848 |
| 700 | -57.130384 | -316.567088 | 51.197105 | 50.383217 | 0.177600 | 0.019848 |
| 701 | -57.151223 | -176.440063 | 29.517711 | 28.081308 | 0.308151 | 0.035611 |
| 702 | -57.151223 | 176.440063 | 29.517711 | 28.081308 | 0.308151 | 0.035611 |
| 703 | -57.334973 | 351.858377 | 56.738596 | 56.000000 | 0.160828 | 0.017857 |
| 704 | -57.797155 | 69.873743 | 14.432160 | 11.120752 | 0.637375 | 0.089922 |
| 705 | -57.797155 | -69.873743 | 14.432160 | 11.120752 | 0.637375 | 0.089922 |
| 706 | -58.238487 | -211.435876 | 34.904261 | 33.651065 | 0.265553 | 0.029717 |
| 707 | -58.238487 | 211.435876 | 34.904261 | 33.651065 | 0.265553 | 0.029717 |
| 708 | -58.985465 | -33.192087 | 10.772097 | 5.282685 | 0.871495 | 0.189298 |
| 709 | -58.985465 | 33.192087 | 10.772097 | 5.282685 | 0.871495 | 0.189298 |
| 710 | -59.167429 | -246.361687 | 40.324620 | 39.209680 | 0.233525 | 0.025504 |
| 711 | -59.167429 | 246.361687 | 40.324620 | 39.209680 | 0.233525 | 0.025504 |
| 712 | -59.256196 | -141.231341 | 24.375965 | 22.477666 | 0.386894 | 0.044489 |
| 713 | -59.256196 | 141.231341 | 24.375965 | 22.477666 | 0.386894 | 0.044489 |
| 714 | -59.966273 | 281.381357 | 45.788914 | 44.783234 | 0.208433 | 0.022330 |
| 715 | -59.966273 | -281.381357 | 45.788914 | 44.783234 | 0.208433 | 0.022330 |
| 716 | -60.004171 | 1.461338 | 9.552792 | 0.232579 | 0.999704 | 4.299611 |
| 717 | -60.004171 | -1.461338 | 9.552792 | 0.232579 | 0.999704 | 4.299611 |
| 718 | -60.524592 | 316.563727 | 51.295275 | 50.382682 | 0.187791 | 0.019848 |
| 719 | -60.524592 | -316.563727 | 51.295275 | 50.382682 | 0.187791 | 0.019848 |
| 720 | -60.724664 | 351.858377 | 56.827855 | 56.000000 | 0.170069 | 0.017857 |
| 721 | -60.725048 | -176.425011 | 29.695649 | 28.078913 | 0.325458 | 0.035614 |
| 722 | -60.725048 | 176.425011 | 29.695649 | 28.078913 | 0.325458 | 0.035614 |
| 723 | -61.050615 | 105.665542 | 19.422371 | 16.817193 | 0.500274 | 0.059463 |
| 724 | -61.050615 | -105.665542 | 19.422371 | 16.817193 | 0.500274 | 0.059463 |
| 725 | -61.075659 | 0.000000 | 9.720493 | 0.000000 | 1.000000 | inf |
| 726 | -61.346469 | 69.792184 | 14.788859 | 11.107771 | 0.660199 | 0.090027 |
| 727 | -61.346469 | -69.792184 | 14.788859 | 11.107771 | 0.660199 | 0.090027 |
| 728 | -61.829886 | -211.392322 | 35.053727 | 33.644133 | 0.280727 | 0.029723 |
| 729 | -61.829886 | 211.392322 | 35.053727 | 33.644133 | 0.280727 | 0.029723 |
| 730 | -62.781634 | 246.294823 | 40.452501 | 39.199039 | 0.247006 | 0.025511 |
| 731 | -62.781634 | -246.294823 | 40.452501 | 39.199039 | 0.247006 | 0.025511 |
| 732 | -62.929241 | 32.946966 | 11.305147 | 5.243673 | 0.885924 | 0.190706 |
| 733 | -62.929241 | -32.946966 | 11.305147 | 5.243673 | 0.885924 | 0.190706 |
| 734 | -63.197906 | 141.166186 | 24.616011 | 22.467296 | 0.408606 | 0.044509 |
| 735 | -63.197906 | -141.166186 | 24.616011 | 22.467296 | 0.408606 | 0.044509 |
| 736 | -63.604162 | 281.320050 | 45.903569 | 44.773477 | 0.220526 | 0.022335 |
| 737 | -63.604162 | -281.320050 | 45.903569 | 44.773477 | 0.220526 | 0.022335 |
| 738 | -64.180338 | -316.529779 | 51.402419 | 50.377279 | 0.198719 | 0.019850 |
| 739 | -64.180338 | 316.529779 | 51.402419 | 50.377279 | 0.198719 | 0.019850 |
| 740 | -64.386941 | 351.858377 | 56.929880 | 56.000000 | 0.180002 | 0.017857 |
| 741 | -64.589799 | 105.551154 | 19.694669 | 16.798988 | 0.521958 | 0.059527 |
| 742 | -64.589799 | -105.551154 | 19.694669 | 16.798988 | 0.521958 | 0.059527 |
| 743 | -64.637285 | 69.696959 | 15.128634 | 11.092615 | 0.679992 | 0.090150 |
| 744 | -64.637285 | -69.696959 | 15.128634 | 11.092615 | 0.679992 | 0.090150 |
| 745 | -64.694697 | -176.381060 | 29.900670 | 28.071918 | 0.344356 | 0.035623 |
| 746 | -64.694697 | 176.381060 | 29.900670 | 28.071918 | 0.344356 | 0.035623 |
| 747 | -64.771747 | 0.000000 | 10.308744 | 0.000000 | 1.000000 | inf |
| 748 | -65.832104 | -211.365674 | 35.233797 | 33.639892 | 0.297371 | 0.029727 |
| 749 | -65.832104 | 211.365674 | 35.233797 | 33.639892 | 0.297371 | 0.029727 |
| 750 | -66.701546 | 141.026543 | 24.828978 | 22.445071 | 0.427560 | 0.044553 |
| 751 | -66.701546 | -141.026543 | 24.828978 | 22.445071 | 0.427560 | 0.044553 |
| 752 | -66.800140 | -246.281319 | 40.613132 | 39.196889 | 0.261777 | 0.025512 |
| 753 | -66.800140 | 246.281319 | 40.613132 | 39.196889 | 0.261777 | 0.025512 |
| 754 | -67.619843 | -281.313309 | 46.047687 | 44.772404 | 0.233715 | 0.022335 |
| 755 | -67.619843 | 281.313309 | 46.047687 | 44.772404 | 0.233715 | 0.022335 |
| 756 | -67.868949 | -105.428356 | 19.955601 | 16.779444 | 0.541286 | 0.059597 |
| 757 | -67.868949 | 105.428356 | 19.955601 | 16.779444 | 0.541286 | 0.059597 |
| 758 | -68.186016 | 316.527115 | 51.532480 | 50.376855 | 0.210588 | 0.019850 |
| 759 | -68.186016 | -316.527115 | 51.532480 | 50.376855 | 0.210588 | 0.019850 |
| 760 | -68.198245 | 176.244217 | 30.076926 | 28.050138 | 0.360878 | 0.035650 |
| 761 | -68.198245 | -176.244217 | 30.076926 | 28.050138 | 0.360878 | 0.035650 |
| 762 | -68.388553 | 351.858377 | 57.047959 | 56.000000 | 0.190793 | 0.017857 |
| 763 | -68.578841 | -32.592349 | 12.084586 | 5.187233 | 0.903189 | 0.192781 |
| 764 | -68.578841 | 32.592349 | 12.084586 | 5.187233 | 0.903189 | 0.192781 |
| 765 | -68.595368 | -69.515583 | 15.543288 | 11.063749 | 0.702380 | 0.090385 |
| 766 | -68.595368 | 69.515583 | 15.543288 | 11.063749 | 0.702380 | 0.090385 |
| 767 | -69.371175 | 211.255741 | 35.388755 | 33.622396 | 0.311985 | 0.029742 |
| 768 | -69.371175 | -211.255741 | 35.388755 | 33.622396 | 0.311985 | 0.029742 |
| 769 | -69.970974 | -140.859652 | 25.032082 | 22.418510 | 0.444878 | 0.044606 |
| 770 | -69.970974 | 140.859652 | 25.032082 | 22.418510 | 0.444878 | 0.044606 |
| 771 | -70.388014 | 246.207763 | 40.755083 | 39.185182 | 0.274876 | 0.025520 |
| 772 | -70.388014 | -246.207763 | 40.755083 | 39.185182 | 0.274876 | 0.025520 |
| 773 | -71.236074 | 281.270855 | 46.179040 | 44.765647 | 0.245513 | 0.022339 |
| 774 | -71.236074 | -281.270855 | 46.179040 | 44.765647 | 0.245513 | 0.022339 |
| 775 | -71.291210 | 3.919085 | 11.363480 | 0.623742 | 0.998492 | 1.603228 |
| 776 | -71.291210 | -3.919085 | 11.363480 | 0.623742 | 0.998492 | 1.603228 |
| 777 | -71.480906 | 176.099249 | 30.248010 | 28.027066 | 0.376109 | 0.035680 |
| 778 | -71.480906 | -176.099249 | 30.248010 | 28.027066 | 0.376109 | 0.035680 |
| 779 | -71.511939 | -32.497012 | 12.501530 | 5.172060 | 0.910407 | 0.193347 |
| 780 | -71.511939 | 32.497012 | 12.501530 | 5.172060 | 0.910407 | 0.193347 |
| 781 | -71.808080 | 316.508402 | 51.654047 | 50.373877 | 0.221253 | 0.019852 |
| 782 | -71.808080 | -316.508402 | 51.654047 | 50.373877 | 0.221253 | 0.019852 |
| 783 | -71.851202 | 105.271767 | 20.285070 | 16.754522 | 0.563738 | 0.059685 |
| 784 | -71.851202 | -105.271767 | 20.285070 | 16.754522 | 0.563738 | 0.059685 |
| 785 | -72.010158 | 351.858377 | 57.160732 | 56.000000 | 0.200501 | 0.017857 |
| 786 | -72.173154 | -32.720681 | 12.612070 | 5.207658 | 0.910772 | 0.192025 |
| 787 | -72.173154 | 32.720681 | 12.612070 | 5.207658 | 0.910772 | 0.192025 |
| 788 | -72.681232 | 211.168850 | 35.543559 | 33.608566 | 0.325448 | 0.029754 |
| 789 | -72.681232 | -211.168850 | 35.543559 | 33.608566 | 0.325448 | 0.029754 |
| 790 | -73.692957 | 246.188140 | 40.899802 | 39.182059 | 0.286764 | 0.025522 |
| 791 | -73.692957 | -246.188140 | 40.899802 | 39.182059 | 0.286764 | 0.025522 |
| 792 | -73.972481 | -2.000937 | 11.777392 | 0.318459 | 0.999634 | 3.140121 |
| 793 | -73.972481 | 2.000937 | 11.777392 | 0.318459 | 0.999634 | 3.140121 |
| 794 | -73.984999 | -140.684708 | 25.298111 | 22.390667 | 0.465453 | 0.044661 |
| 795 | -73.984999 | 140.684708 | 25.298111 | 22.390667 | 0.465453 | 0.044661 |
| 796 | -74.508606 | -281.292379 | 46.312977 | 44.769073 | 0.256049 | 0.022337 |
| 797 | -74.508606 | 281.292379 | 46.312977 | 44.769073 | 0.256049 | 0.022337 |
| 798 | -74.757158 | 69.289437 | 16.222612 | 11.027756 | 0.733419 | 0.090680 |
| 799 | -74.757158 | -69.289437 | 16.222612 | 11.027756 | 0.733419 | 0.090680 |
| 800 | -75.045687 | -316.530852 | 51.773970 | 50.377450 | 0.230693 | 0.019850 |
| 801 | -75.045687 | 316.530852 | 51.773970 | 50.377450 | 0.230693 | 0.019850 |
| 802 | -75.233144 | 351.858377 | 57.265785 | 56.000000 | 0.209090 | 0.017857 |
| 803 | -75.487405 | 175.907873 | 30.465569 | 27.996608 | 0.394353 | 0.035719 |
| 804 | -75.487405 | -175.907873 | 30.465569 | 27.996608 | 0.394353 | 0.035719 |
| 805 | -76.676467 | -211.007198 | 35.731372 | 33.582839 | 0.341533 | 0.029777 |
| 806 | -76.676467 | 211.007198 | 35.731372 | 33.582839 | 0.341533 | 0.029777 |
| 807 | -77.232425 | 69.389625 | 16.524367 | 11.043702 | 0.743866 | 0.090549 |
| 808 | -77.232425 | -69.389625 | 16.524367 | 11.043702 | 0.743866 | 0.090549 |
| 809 | -77.356873 | -69.260283 | 16.525367 | 11.023116 | 0.745020 | 0.090718 |
| 810 | -77.356873 | 69.260283 | 16.525367 | 11.023116 | 0.745020 | 0.090718 |
| 811 | -77.358540 | -32.280256 | 13.340905 | 5.137562 | 0.922875 | 0.194645 |
| 812 | -77.358540 | 32.280256 | 13.340905 | 5.137562 | 0.922875 | 0.194645 |
| 813 | -77.690399 | 246.077645 | 41.069996 | 39.164474 | 0.301067 | 0.025533 |
| 814 | -77.690399 | -246.077645 | 41.069996 | 39.164474 | 0.301067 | 0.025533 |
| 815 | -78.253904 | 105.127152 | 20.858038 | 16.731506 | 0.597108 | 0.059767 |
| 816 | -78.253904 | -105.127152 | 20.858038 | 16.731506 | 0.597108 | 0.059767 |
| 817 | -78.514848 | -281.228183 | 46.470483 | 44.758856 | 0.268902 | 0.022342 |
| 818 | -78.514848 | 281.228183 | 46.470483 | 44.758856 | 0.268902 | 0.022342 |
| 819 | -79.058786 | 316.501686 | 51.920531 | 50.372808 | 0.242343 | 0.019852 |
| 820 | -79.058786 | -316.501686 | 51.920531 | 50.372808 | 0.242343 | 0.019852 |
| 821 | -79.248414 | 351.858377 | 57.402806 | 56.000000 | 0.219724 | 0.017857 |
| 822 | -80.292059 | -105.140847 | 21.055070 | 16.733686 | 0.606926 | 0.059760 |
| 823 | -80.292059 | 105.140847 | 21.055070 | 16.733686 | 0.606926 | 0.059760 |
| 824 | -80.376065 | -140.569414 | 25.771344 | 22.372317 | 0.496375 | 0.044698 |
| 825 | -80.376065 | 140.569414 | 25.771344 | 22.372317 | 0.496375 | 0.044698 |
| 826 | -80.738344 | -105.068490 | 21.089122 | 16.722169 | 0.609314 | 0.059801 |
| 827 | -80.738344 | 105.068490 | 21.089122 | 16.722169 | 0.609314 | 0.059801 |
| 828 | -81.728740 | 175.741308 | 30.846755 | 27.970098 | 0.421682 | 0.035752 |
| 829 | -81.728740 | -175.741308 | 30.846755 | 27.970098 | 0.421682 | 0.035752 |
| 830 | -82.328091 | -4.824289 | 13.125400 | 0.767809 | 0.998288 | 1.302406 |
| 831 | -82.328091 | 4.824289 | 13.125400 | 0.767809 | 0.998288 | 1.302406 |
| 832 | -82.600442 | 140.579658 | 25.950297 | 22.373948 | 0.506594 | 0.044695 |
| 833 | -82.600442 | -140.579658 | 25.950297 | 22.373948 | 0.506594 | 0.044695 |
| 834 | -82.777733 | -69.111821 | 17.162628 | 10.999488 | 0.767626 | 0.090913 |
| 835 | -82.777733 | 69.111821 | 17.162628 | 10.999488 | 0.767626 | 0.090913 |
| 836 | -82.862711 | 210.764530 | 36.043558 | 33.544217 | 0.365891 | 0.029811 |
| 837 | -82.862711 | -210.764530 | 36.043558 | 33.544217 | 0.365891 | 0.029811 |
| 838 | -82.995671 | 140.490869 | 25.970052 | 22.359816 | 0.508631 | 0.044723 |
| 839 | -82.995671 | -140.490869 | 25.970052 | 22.359816 | 0.508631 | 0.044723 |
| 840 | -84.019349 | 245.657331 | 41.321103 | 39.097579 | 0.323614 | 0.025577 |
| 841 | -84.019349 | -245.657331 | 41.321103 | 39.097579 | 0.323614 | 0.025577 |
| 842 | -84.263147 | 25.881773 | 14.029257 | 4.119212 | 0.955923 | 0.242765 |
| 843 | -84.263147 | -25.881773 | 14.029257 | 4.119212 | 0.955923 | 0.242765 |
| 844 | -84.334363 | 3.013423 | 13.430797 | 0.479601 | 0.999362 | 2.085066 |
| 845 | -84.334363 | -3.013423 | 13.430797 | 0.479601 | 0.999362 | 2.085066 |
| 846 | -84.417542 | -175.895762 | 31.051794 | 27.994680 | 0.432679 | 0.035721 |
| 847 | -84.417542 | 175.895762 | 31.051794 | 27.994680 | 0.432679 | 0.035721 |
| 848 | -84.577439 | -175.688159 | 31.033040 | 27.961639 | 0.433761 | 0.035763 |
| 849 | -84.577439 | 175.688159 | 31.033040 | 27.961639 | 0.433761 | 0.035763 |
| 850 | -85.105900 | 280.538672 | 46.658453 | 44.649116 | 0.290302 | 0.022397 |
| 851 | -85.105900 | -280.538672 | 46.658453 | 44.649116 | 0.290302 | 0.022397 |
| 852 | -85.168644 | 38.197052 | 14.855827 | 6.079250 | 0.912437 | 0.164494 |
| 853 | -85.168644 | -38.197052 | 14.855827 | 6.079250 | 0.912437 | 0.164494 |
| 854 | -85.665558 | 211.188899 | 36.271736 | 33.611757 | 0.375888 | 0.029751 |
| 855 | -85.665558 | -211.188899 | 36.271736 | 33.611757 | 0.375888 | 0.029751 |
| 856 | -85.756665 | -210.815246 | 36.222096 | 33.552289 | 0.376803 | 0.029804 |
| 857 | -85.756665 | 210.815246 | 36.222096 | 33.552289 | 0.376803 | 0.029804 |
| 858 | -85.966733 | -315.560139 | 52.053273 | 50.222956 | 0.262847 | 0.019911 |
| 859 | -85.966733 | 315.560139 | 52.053273 | 50.222956 | 0.262847 | 0.019911 |
| 860 | -85.974527 | 105.007973 | 21.599556 | 16.712538 | 0.633498 | 0.059835 |
| 861 | -85.974527 | -105.007973 | 21.599556 | 16.712538 | 0.633498 | 0.059835 |
| 862 | -86.375776 | 246.597853 | 41.585233 | 39.247267 | 0.330577 | 0.025479 |
| 863 | -86.375776 | -246.597853 | 41.585233 | 39.247267 | 0.330577 | 0.025479 |
| 864 | -86.519761 | 350.775137 | 57.500737 | 55.827597 | 0.239476 | 0.017912 |
| 865 | -86.519761 | -350.775137 | 57.500737 | 55.827597 | 0.239476 | 0.017912 |
| 866 | -86.708900 | 282.092865 | 46.969538 | 44.896474 | 0.293811 | 0.022273 |
| 867 | -86.708900 | -282.092865 | 46.969538 | 44.896474 | 0.293811 | 0.022273 |
| 868 | -86.734242 | -245.973742 | 41.510438 | 39.147937 | 0.332547 | 0.025544 |
| 869 | -86.734242 | 245.973742 | 41.510438 | 39.147937 | 0.332547 | 0.025544 |
| 870 | -86.757772 | -317.567748 | 52.394664 | 50.542477 | 0.263537 | 0.019785 |
| 871 | -86.757772 | 317.567748 | 52.394664 | 50.542477 | 0.263537 | 0.019785 |
| 872 | -87.524583 | 281.194311 | 46.871277 | 44.753465 | 0.297196 | 0.022345 |
| 873 | -87.524583 | -281.194311 | 46.871277 | 44.753465 | 0.297196 | 0.022345 |
| 874 | -88.040412 | -316.496355 | 52.284532 | 50.371959 | 0.267996 | 0.019852 |
| 875 | -88.040412 | 316.496355 | 52.284532 | 50.371959 | 0.267996 | 0.019852 |
| 876 | -88.170415 | 140.558523 | 26.407599 | 22.370584 | 0.531391 | 0.044702 |
| 877 | -88.170415 | -140.558523 | 26.407599 | 22.370584 | 0.531391 | 0.044702 |
| 878 | -88.219075 | 351.858377 | 57.733315 | 56.000000 | 0.243196 | 0.017857 |
| 879 | -88.657831 | 27.265548 | 14.762529 | 4.339447 | 0.955821 | 0.230444 |
| 880 | -88.657831 | -27.265548 | 14.762529 | 4.339447 | 0.955821 | 0.230444 |
| 881 | -89.526558 | 36.237090 | 15.371543 | 5.767312 | 0.926946 | 0.173391 |
| 882 | -89.526558 | -36.237090 | 15.371543 | 5.767312 | 0.926946 | 0.173391 |
| 883 | -89.792477 | 175.890513 | 31.430648 | 27.993845 | 0.454681 | 0.035722 |
| 884 | -89.792477 | -175.890513 | 31.430648 | 27.993845 | 0.454681 | 0.035722 |
| 885 | -90.026130 | -62.325176 | 17.426653 | 9.919360 | 0.822195 | 0.100813 |
| 886 | -90.026130 | 62.325176 | 17.426653 | 9.919360 | 0.822195 | 0.100813 |
| 887 | -90.376140 | 75.533052 | 18.745918 | 12.021459 | 0.767304 | 0.083185 |
| 888 | -90.376140 | -75.533052 | 18.745918 | 12.021459 | 0.767304 | 0.083185 |
| 889 | -90.531744 | 0.000000 | 14.408575 | 0.000000 | 1.000000 | inf |
| 890 | -91.010852 | -211.042064 | 36.578546 | 33.588388 | 0.395992 | 0.029772 |
| 891 | -91.010852 | 211.042064 | 36.578546 | 33.588388 | 0.395992 | 0.029772 |
| 892 | -91.898481 | -246.139516 | 41.815668 | 39.174321 | 0.349776 | 0.025527 |
| 893 | -91.898481 | 246.139516 | 41.815668 | 39.174321 | 0.349776 | 0.025527 |
| 894 | -92.541781 | -281.308683 | 47.132053 | 44.771668 | 0.312494 | 0.022336 |
| 895 | -92.541781 | 281.308683 | 47.132053 | 44.771668 | 0.312494 | 0.022336 |
| 896 | -92.956744 | -316.560408 | 52.509422 | 50.382154 | 0.281750 | 0.019848 |
| 897 | -92.956744 | 316.560408 | 52.509422 | 50.382154 | 0.281750 | 0.019848 |
| 898 | -93.101793 | 351.858377 | 57.927209 | 56.000000 | 0.255797 | 0.017857 |
| 899 | -93.387365 | -98.033026 | 21.548706 | 15.602441 | 0.689743 | 0.064093 |
| 900 | -93.387365 | 98.033026 | 21.548706 | 15.602441 | 0.689743 | 0.064093 |
| 901 | -93.532091 | -111.580776 | 23.172502 | 17.758632 | 0.642403 | 0.056311 |
| 902 | -93.532091 | 111.580776 | 23.172502 | 17.758632 | 0.642403 | 0.056311 |
| 903 | -93.692886 | -4.289078 | 14.927302 | 0.682628 | 0.998954 | 1.464927 |
| 904 | -93.692886 | 4.289078 | 14.927302 | 0.682628 | 0.998954 | 1.464927 |
| 905 | -94.349268 | 63.861696 | 18.132562 | 10.163905 | 0.828132 | 0.098387 |
| 906 | -94.349268 | -63.861696 | 18.132562 | 10.163905 | 0.828132 | 0.098387 |
| 907 | -94.745043 | 73.683778 | 19.102520 | 11.727138 | 0.789380 | 0.085272 |
| 908 | -94.745043 | -73.683778 | 19.102520 | 11.727138 | 0.789380 | 0.085272 |
| 909 | -95.202577 | 6.142057 | 15.183461 | 0.977539 | 0.997925 | 1.022977 |
| 910 | -95.202577 | -6.142057 | 15.183461 | 0.977539 | 0.997925 | 1.022977 |
| 911 | -95.692689 | 133.532122 | 26.145974 | 21.252297 | 0.582497 | 0.047054 |
| 912 | -95.692689 | -133.532122 | 26.145974 | 21.252297 | 0.582497 | 0.047054 |
| 913 | -95.747216 | -147.119968 | 27.936936 | 23.414870 | 0.545466 | 0.042708 |
| 914 | -95.747216 | 147.119968 | 27.936936 | 23.414870 | 0.545466 | 0.042708 |
| 915 | -97.319856 | 168.949269 | 31.031137 | 26.889111 | 0.499142 | 0.037190 |
| 916 | -97.319856 | -168.949269 | 31.031137 | 26.889111 | 0.499142 | 0.037190 |
| 917 | -97.471196 | 182.439238 | 32.920349 | 29.036107 | 0.471229 | 0.034440 |
| 918 | -97.471196 | -182.439238 | 32.920349 | 29.036107 | 0.471229 | 0.034440 |
| 919 | -97.628457 | -99.626238 | 22.200091 | 15.856008 | 0.699909 | 0.063068 |
| 920 | -97.628457 | 99.626238 | 22.200091 | 15.856008 | 0.699909 | 0.063068 |
| 921 | -97.948677 | -19.505992 | 15.895131 | 3.104475 | 0.980742 | 0.322116 |
| 922 | -97.948677 | 19.505992 | 15.895131 | 3.104475 | 0.980742 | 0.322116 |
| 923 | -97.952152 | -109.843672 | 23.423508 | 17.482163 | 0.665552 | 0.057201 |
| 924 | -97.952152 | 109.843672 | 23.423508 | 17.482163 | 0.665552 | 0.057201 |
| 925 | -98.399975 | 204.263076 | 36.085013 | 32.509478 | 0.433999 | 0.030760 |
| 926 | -98.399975 | -204.263076 | 36.085013 | 32.509478 | 0.433999 | 0.030760 |
| 927 | -98.873793 | -217.775410 | 38.065044 | 34.660033 | 0.413404 | 0.028852 |
| 928 | -98.873793 | 217.775410 | 38.065044 | 34.660033 | 0.413404 | 0.028852 |
| 929 | -99.104578 | -239.370236 | 41.233058 | 38.096956 | 0.382532 | 0.026249 |
| 930 | -99.104578 | 239.370236 | 41.233058 | 38.096956 | 0.382532 | 0.026249 |
| 931 | -99.299795 | -43.283124 | 17.240145 | 6.888723 | 0.916701 | 0.145165 |
| 932 | -99.299795 | 43.283124 | 17.240145 | 6.888723 | 0.916701 | 0.145165 |
| 933 | -99.680943 | -274.334108 | 46.454570 | 43.661629 | 0.341510 | 0.022903 |
| 934 | -99.680943 | 274.334108 | 46.454570 | 43.661629 | 0.341510 | 0.022903 |
| 935 | -99.833988 | -135.101920 | 26.735830 | 21.502138 | 0.594299 | 0.046507 |
| 936 | -99.833988 | 135.101920 | 26.735830 | 21.502138 | 0.594299 | 0.046507 |
| 937 | -99.860702 | 253.218384 | 43.321645 | 40.300958 | 0.366868 | 0.024813 |
| 938 | -99.860702 | -253.218384 | 43.321645 | 40.300958 | 0.366868 | 0.024813 |
| 939 | -100.175662 | -309.342071 | 51.750492 | 49.233320 | 0.308083 | 0.020311 |
| 940 | -100.175662 | 309.342071 | 51.750492 | 49.233320 | 0.308083 | 0.020311 |
| 941 | -100.185518 | -145.532934 | 28.120014 | 23.162286 | 0.567035 | 0.043174 |
| 942 | -100.185518 | 145.532934 | 28.120014 | 23.162286 | 0.567035 | 0.043174 |
| 943 | -100.392397 | 288.649950 | 48.639330 | 45.940066 | 0.328498 | 0.021767 |
| 944 | -100.392397 | -288.649950 | 48.639330 | 45.940066 | 0.328498 | 0.021767 |
| 945 | -100.497591 | -344.475254 | 57.110455 | 54.824939 | 0.280066 | 0.018240 |
| 946 | -100.497591 | 344.475254 | 57.110455 | 54.824939 | 0.280066 | 0.018240 |
| 947 | -100.581039 | 323.994069 | 53.992878 | 51.565258 | 0.296483 | 0.019393 |
| 948 | -100.581039 | -323.994069 | 53.992878 | 51.565258 | 0.296483 | 0.019393 |
| 949 | -101.426917 | 170.408514 | 31.561866 | 27.121357 | 0.511459 | 0.036871 |
| 950 | -101.426917 | -170.408514 | 31.561866 | 27.121357 | 0.511459 | 0.036871 |
| 951 | -101.798814 | 180.982879 | 33.048247 | 28.804320 | 0.490246 | 0.034717 |
| 952 | -101.798814 | -180.982879 | 33.048247 | 28.804320 | 0.490246 | 0.034717 |
| 953 | -101.883732 | 6.664475 | 16.249954 | 1.060684 | 0.997867 | 0.942788 |
| 954 | -101.883732 | -6.664475 | 16.249954 | 1.060684 | 0.997867 | 0.942788 |
| 955 | -102.388876 | 20.965247 | 16.633804 | 3.336723 | 0.979673 | 0.299695 |
| 956 | -102.388876 | -20.965247 | 16.633804 | 3.336723 | 0.979673 | 0.299695 |
| 957 | -102.649996 | -205.587598 | 36.572158 | 32.720283 | 0.446713 | 0.030562 |
| 958 | -102.649996 | 205.587598 | 36.572158 | 32.720283 | 0.446713 | 0.030562 |
| 959 | -102.964960 | -216.303713 | 38.127187 | 34.425805 | 0.429808 | 0.029048 |
| 960 | -102.964960 | 216.303713 | 38.127187 | 34.425805 | 0.429808 | 0.029048 |
| 961 | -103.611476 | 240.711782 | 41.708769 | 38.310470 | 0.395367 | 0.026103 |
| 962 | -103.611476 | -240.711782 | 41.708769 | 38.310470 | 0.395367 | 0.026103 |
| 963 | -103.754665 | -56.004045 | 18.765092 | 8.913321 | 0.879989 | 0.112192 |
| 964 | -103.754665 | 56.004045 | 18.765092 | 8.913321 | 0.879989 | 0.112192 |
| 965 | -103.791363 | 4.588853 | 16.535046 | 0.730339 | 0.999024 | 1.369228 |
| 966 | -103.791363 | -4.588853 | 16.535046 | 0.730339 | 0.999024 | 1.369228 |
| 967 | -103.792027 | 41.452110 | 17.787701 | 6.597308 | 0.928676 | 0.151577 |
| 968 | -103.792027 | -41.452110 | 17.787701 | 6.597308 | 0.928676 | 0.151577 |
| 969 | -103.817204 | -251.551913 | 43.311314 | 40.035730 | 0.381494 | 0.024978 |
| 970 | -103.817204 | 251.551913 | 43.311314 | 40.035730 | 0.381494 | 0.024978 |
| 971 | -103.986268 | -34.363132 | 17.430169 | 5.469062 | 0.949499 | 0.182847 |
| 972 | -103.986268 | 34.363132 | 17.430169 | 5.469062 | 0.949499 | 0.182847 |
| 973 | -104.260819 | -80.880610 | 21.001212 | 12.872549 | 0.790127 | 0.077685 |
| 974 | -104.260819 | 80.880610 | 21.001212 | 12.872549 | 0.790127 | 0.077685 |
| 975 | -104.322107 | 275.875236 | 46.941333 | 43.906907 | 0.353705 | 0.022775 |
| 976 | -104.322107 | -275.875236 | 46.941333 | 43.906907 | 0.353705 | 0.022775 |
| 977 | -104.432405 | -286.781615 | 48.574814 | 45.642712 | 0.342172 | 0.021909 |
| 978 | -104.432405 | 286.781615 | 48.574814 | 45.642712 | 0.342172 | 0.021909 |
| 979 | -104.765916 | -311.109362 | 52.246700 | 49.514593 | 0.319140 | 0.020196 |
| 980 | -104.765916 | 311.109362 | 52.246700 | 49.514593 | 0.319140 | 0.020196 |
| 981 | -104.812659 | -322.040367 | 53.900610 | 51.254316 | 0.309485 | 0.019511 |
| 982 | -104.812659 | 322.040367 | 53.900610 | 51.254316 | 0.309485 | 0.019511 |
| 983 | -104.929967 | 346.389833 | 57.603584 | 55.129654 | 0.289915 | 0.018139 |
| 984 | -104.929967 | -346.389833 | 57.603584 | 55.129654 | 0.289915 | 0.018139 |
| 985 | -105.208237 | 0.000000 | 16.744411 | 0.000000 | 1.000000 | inf |
| 986 | -106.069332 | 34.680255 | 17.760881 | 5.519534 | 0.950485 | 0.181175 |
| 987 | -106.069332 | -34.680255 | 17.760881 | 5.519534 | 0.950485 | 0.181175 |
| 988 | -106.442343 | -69.300672 | 20.214906 | 11.029544 | 0.838036 | 0.090666 |
| 989 | -106.442343 | 69.300672 | 20.214906 | 11.029544 | 0.838036 | 0.090666 |
| 990 | -107.170073 | 91.634457 | 22.441580 | 14.584077 | 0.760047 | 0.068568 |
| 991 | -107.170073 | -91.634457 | 22.441580 | 14.584077 | 0.760047 | 0.068568 |
| 992 | -107.393409 | 117.085123 | 25.286245 | 18.634676 | 0.675948 | 0.053663 |
| 993 | -107.393409 | -117.085123 | 25.286245 | 18.634676 | 0.675948 | 0.053663 |
| 994 | -108.092648 | 57.766084 | 19.506022 | 9.193758 | 0.881957 | 0.108769 |
| 995 | -108.092648 | -57.766084 | 19.506022 | 9.193758 | 0.881957 | 0.108769 |
| 996 | -108.203753 | 32.422115 | 17.977638 | 5.160140 | 0.957921 | 0.193793 |
| 997 | -108.203753 | -32.422115 | 17.977638 | 5.160140 | 0.957921 | 0.193793 |
| 998 | -108.324174 | 69.398551 | 20.474951 | 11.045122 | 0.842020 | 0.090538 |
| 999 | -108.324174 | -69.398551 | 20.474951 | 11.045122 | 0.842020 | 0.090538 |
Generating an instance of StabilityDerivatives
Variable print_info has no assigned value in the settings file.
will default to the value: c_bool(True)
Variable folder has no assigned value in the settings file.
will default to the value: ./output/
/home/ng213/code/sharpy/sharpy/postproc/asymptoticstability.py:171: UserWarning: Plotting modes is under development
warn.warn('Plotting modes is under development')
|==============|==============|==============|==============|==============|==============|==============|
| der | X | Y | Z | L | M | N |
|==============|==============|==============|==============|==============|==============|==============|
| u | -0.000000 | -0.032063 | 0.000000 | 103.702544 | -0.000000 | 0.215455 |
| v | 131.843986 | 0.000000 | -982.084813 | -0.000000 | 1279.474176 | -0.000000 |
| w | 0.000000 | -187.481605 | -0.000000 |-22078.785295 | 0.000000 | -3334.924961 |
| p | -187.422614 | 0.000000 | 1572.771760 | 0.000000 | -2857.778575 | 0.000000 |
| q | 0.000000 | -2.610892 | 0.000000 | 1127.543337 | -0.000000 | -38.871906 |
| r | 0.000000 | 0.000000 | -0.000000 | 0.000000 | 0.000000 | 0.000000 |
| flap1 | -0.000000 | 1.108474 | 0.000000 | 271.384778 | -0.000000 | 23.331888 |
|==============|==============|==============|==============|==============|==============|==============|
| der | C_D | C_Y | C_L | C_l | C_m | C_n |
|==============|==============|==============|==============|==============|==============|==============|
| u | -0.000000 | -0.000006 | 0.000000 | 0.001009 | -0.000000 | -0.000078 |
| v | 0.010573 | 0.000000 | -0.193187 | -0.000000 | 0.347457 | -0.000000 |
| w | 0.000000 | -0.036606 | -0.000000 | -0.217442 | 0.000000 | -0.015495 |
| p | -0.479599 | 0.000000 | 12.034020 | 0.000000 | -30.222290 | 0.000000 |
| q | 0.000000 | -0.019853 | 0.000000 | 0.010944 | -0.000000 | -0.001245 |
| r | 0.000000 | 0.000000 | -0.000000 | 0.000000 | 0.000000 | 0.000000 |
| alpha | 0.000000 | -1.024980 | -0.000000 | -6.088362 | 0.000000 | -0.433847 |
| beta | 0.296049 | 0.000000 | -5.409222 | -0.000000 | 9.728798 | -0.000000 |
FINISHED - Elapsed time = 29.3233356 seconds
FINISHED - CPU process time = 69.2634336 seconds
Post-processing¶
Nonlinear Equilibrium¶
The files can be opened with Paraview to see the deformation and aerodynamic loading on the flying wing in trim conditions.
Asymptotic Stability¶
[18]:
eigenvalues_trim = np.loadtxt('./output/horten_u_inf2800_M4N11Msf5/stability/eigenvalues.dat')
Flight Dynamics modes¶
The flight dynamics modes can be found close to the origin of the Argand diagram. In particular, the phugoid is the mode that is closest to the imaginary axis. An exercise is left to the user to compare this phugoid predicition with the nonlinear response!
[19]:
fig = plt.figure()
plt.scatter(eigenvalues_trim[:, 0], eigenvalues_trim[:, 1],
marker='x',
color='k')
plt.xlim(-0.5, 0.5)
plt.ylim(-0.5, 0.5)
plt.grid()
plt.xlabel('Real Part, $Re(\lambda)$ [rad/s]')
plt.ylabel('Imaginary Part, $Im(\lambda)$ [rad/s]');
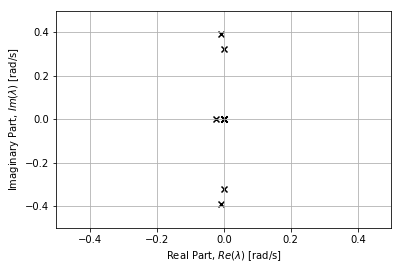
Structural Modes¶
Looking further out on the plot, the structural modes appear. There is a curve given the Newmark-\(\beta\) integration scheme and on top of it several modes are damped by the presence of the aerodynamics.
Try changing newmark_damp
in the LinearAssembler
settings to see how this plot changes!
[20]:
fig = plt.figure()
plt.scatter(eigenvalues_trim[:, 0], eigenvalues_trim[:, 1],
marker='x',
color='k')
plt.xlim(-5, 0.5)
plt.ylim(-200, 200)
plt.grid()
plt.xlabel('Real Part, $Re(\lambda)$ [rad/s]')
plt.ylabel('Imaginary Part, $Im(\lambda)$ [rad/s]');
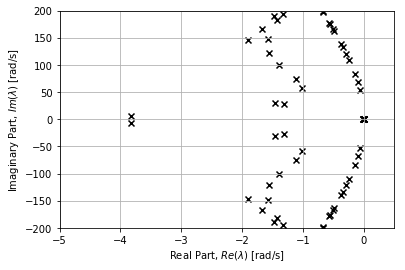
Stability Derivatives¶
Stability derivatives are calculated using the steady-state frequency response of the UVLM system. The output is saved in ./output/<case_name>/stability/
. Note that stability derivatives are expressed in the SHARPy Frame of Reference, which is South East Up (not the conventional in flight dynamics literature).
This body attached frame of reference has:
\(x\) positive downstream
\(y\) positive towards the right wing
\(z\) positive up